How to connect to an API using Python?
Connecting to an API using Python is a common task when integrating third-party services or accessing data. The process generally involves sending HTTP requests and handling the responses.
- Using the `requests` library:
The `requests` library is one of the most popular tools in Python for making HTTP requests. It abstracts the complexities of making requests behind a simple API, providing methods for functionalities like passing parameters or handling cookies.
Example:
```python import requests url = "https://api.example.com/data" response = requests.get(url) if response.status_code == 200: data = response.json() else: print(f"Failed to retrieve data. Status code: {response.status_code}") ```
- Authentication:
Many APIs require authentication. The `requests` library easily handles different types of authentication methods.
– Basic Authentication:
```python response = requests.get(url, auth=('username', 'password')) ```
– Bearer Token (OAuth2):
```python headers = {"Authorization": "Bearer YOUR_ACCESS_TOKEN"} response = requests.get(url, headers=headers) ```
- Error Handling:
Always ensure to handle potential errors that might arise during the API call. This includes checking the status code, timeouts, or exceptions.
- Rate Limiting:
Some APIs have rate limits, meaning you can only make a certain number of requests in a given timeframe. Always read the API documentation to understand its rate limits, and consider using tools or libraries that help manage these limits by adding delays or managing retries.
- Conclusion:
Python, with its `requests` library, provides a straightforward way to connect to APIs. Always ensure to understand the API’s documentation, handle potential errors gracefully, and respect the API’s usage policies and rate limits. By following best practices, you can efficiently and safely interact with any API from your Python applications.
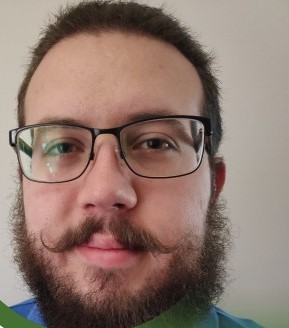
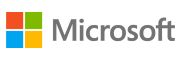