The Art of Converting Data Types: A Python Function Tutorial
Python, as a dynamically typed language, allows flexibility in data types. However, when dealing with complex data, there might be times where you need to explicitly convert data types. Understanding this process is important not only for your personal programming skills but also if you plan to hire Python developers for more intricate tasks. This article is designed to guide you on how to use Python’s in-built functions to transform data types, thereby helping you build your understanding and enabling you to set clearer expectations when you hire Python developers.
Understanding Python’s Data Types
Before we delve into the conversion process, let’s take a quick overview of Python’s basic data types:
- Integer (`int`): These are whole numbers which can be positive or negative. E.g., 5, -7, 39.
- Float (`float`): These are real numbers that contain a decimal point. E.g., 3.14, -0.7, 8.1.
- Boolean (`bool`): This type has two values – True or False, often used in logical operations.
- String (`str`): This is a sequence of Unicode characters. E.g., “Hello, World!”
- List (`list`): This is an ordered collection of items which can be of different data types.
- Tuple (`tuple`): Similar to a list but immutable (cannot be changed once defined).
- Dictionary (`dict`): An unordered collection of data stored as key-value pairs.
Conversion Functions
Python provides a suite of functions to convert between these types:
– `int()`: Converts a value to an integer.
– `float()`: Converts a value to a floating-point number.
– `str()`: Converts a value to a string.
– `bool()`: Converts a value to a Boolean.
– `list()`: Converts a value to a list.
– `tuple()`: Converts a value to a tuple.
– `dict()`: Converts a value to a dictionary.
Now, let’s take a look at each of these functions in detail.
Converting to Integer
In Python, the `int()` function converts a number or a string to an integer. Let’s see it in action:
```python print(int(3.14)) # Output: 3 print(int("10")) # Output: 10 ```
This function works well with strings that can be interpreted as integers. However, if you try to convert a string that doesn’t represent an integer, you’ll get a `ValueError`. For example, `int(“hello”)` will cause an error.
Converting to Float
The `float()` function in Python converts integers and strings to floating-point numbers:
```python print(float(3)) # Output: 3.0 print(float("3.14")) # Output: 3.14 ```
Just like with `int()`, trying to convert a non-numeric string to a float will cause a `ValueError`.
Converting to String
The `str()` function converts other data types to strings. This function can come in handy when you want to concatenate a number with a string:
```python print(str(3.14)) # Output: '3.14' print(str(True)) # Output: 'True' ```
Converting to Boolean
The `bool()` function converts a value to a Boolean value (`True` or `False`). By default, Python considers the following as `False`:
– None
– False
– Zero of any numeric type, e.g., 0, 0.0.
– Empty sequences and collections, e.g., ”, (), [].
All other values are considered `True`.
```python print(bool(0)) # Output: False print(bool(3.14)) # Output: True ```
Converting to List, Tuple, and Dictionary
The `list()`, `tuple()`, and `dict()` functions convert values to a list, tuple, and dictionary, respectively. Here’s how you can use them:
```python print(list("hello")) # Output: ['h', 'e', 'l', 'l', 'o'] print(tuple([1, 2, 3])) # Output: (1, 2, 3) print(dict([(1, 'one'), (2, 'two')])) # Output: {1: 'one', 2: 'two'} ```
Note: `dict()` requires each item to be a pair.
Important Considerations
While Python’s data type conversion functions are straightforward, remember these crucial points:
– Not all conversions are possible. For instance, you can’t convert a non-numeric string into an integer or a float.
– Conversions can lead to data loss. For example, converting a float to an integer discards the decimal part.
– Python is zero-indexed. When converting sequences (like strings) into lists or tuples, the first item is at index 0.
Conclusion
Mastering data type conversion in Python not only allows you to manipulate data more effectively, but also opens the door to advanced data analysis and manipulation. These skills are essential when looking to hire Python developers, as this proficiency demonstrates a deep understanding of data handling. Gaining knowledge of these built-in Python functions can help you clean and preprocess data, which is a critical step in data analysis and machine learning. By understanding these Python functions, you’re not just improving your own skills but also setting the standard to hire Python developers. Happy coding!
Table of Contents
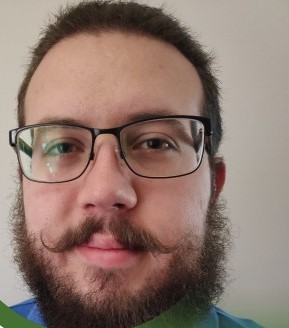
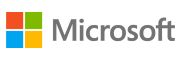