Python Q & A
How to convert a string to an integer in Python?
Converting data types is a common operation in programming, and in Python, turning a string into an integer is a straightforward task. Here’s how you can achieve this:
Using the `int()` Function:
The primary method to convert a string to an integer in Python is to use the built-in `int()` function.
```python number_string = "123" converted_integer = int(number_string) print(converted_integer) # Output: 123 ```
However, there are some considerations and potential pitfalls to be aware of:
- Valid Integer Strings: The string must represent a valid integer; otherwise, a `ValueError` will be raised. For instance, trying to convert the string “123.45” or “abc” using `int()` would lead to an error.
```python invalid_string = "123.45" # This will raise a ValueError converted_integer = int(invalid_string) ```
- Base Argument: The `int()` function also accepts an optional second argument, the `base`, which indicates the numeral system of the string. For example, to convert a binary string to an integer, you would use:
```python binary_string = "1101" converted_integer = int(binary_string, 2) print(converted_integer) # Output: 13 ```
- Whitespace: Strings containing numbers with leading or trailing whitespaces are still convertible. The `int()` function will automatically strip the whitespace.
```python whitespace_string = " 42 " converted_integer = int(whitespace_string) print(converted_integer) # Output: 42 ```
- Floating Point Strings: If you have a string that represents a floating-point number and you want to convert it to an integer, you should first convert it to a float and then to an integer. This two-step conversion ensures that the number is rounded down.
```python float_string = "123.45" converted_integer = int(float(float_string)) print(converted_integer) # Output: 123 ```
While converting a string to an integer in Python is a straightforward process using the `int()` function, it’s essential to be aware of the string’s format and content to ensure a successful and error-free conversion.
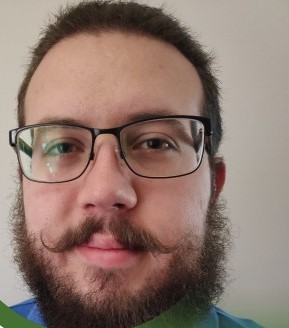
Previously at
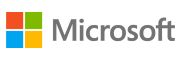
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git