Python Q & A
How to create and use Python packages?
Creating and using Python packages is essential for modular and maintainable code. Here’s a concise guide on how to go about it:
Creating a Python Package:
- Directory Structure: At the heart of a Python package is its directory structure. Start by creating a directory for your package. Let’s call it `mypackage`. Inside this directory, create an empty file named `__init__.py`. This file makes Python treat the directory as a package or a module.
```plaintext mypackage/ |-- __init__.py ```
- Adding Modules: You can now add Python files (modules) to this directory. For instance, `module1.py` inside the `mypackage` directory can be imported using `from mypackage import module1`.
- Nested Packages: For larger packages, you might want to have sub-packages. Just create a new directory inside your main package and add an `__init__.py` file to it.
Using a Python Package:
- Local Import: If your package is in the same directory as your script or is in the Python path, you can import it directly using `import mypackage`.
- Installing with pip: If you’ve uploaded your package to the Python Package Index (PyPI), you or others can install it using `pip install mypackage` and then import it in any script.
Distributing Your Package:
- Setup.py: To distribute your package, create a `setup.py` at the root level. This file contains metadata about your package like its name and version.
- Building and Uploading: Use `setuptools` and `wheel` to build your package and `twine` to upload it to PyPI. First, generate distribution archives with `python setup.py sdist bdist_wheel`, then upload using `twine upload dist/*`.
Best Practices:
– Keep your package responsibilities clear and focused. A package should ideally have a single responsibility.
– Document your package, detailing its functionalities and how other developers can use it.
– Ensure version control for your package to manage updates efficiently.
Creating and using Python packages is about organizing your codebase, making it reusable, and potentially sharing it with the world. Proper structuring and understanding the distribution mechanisms are key.
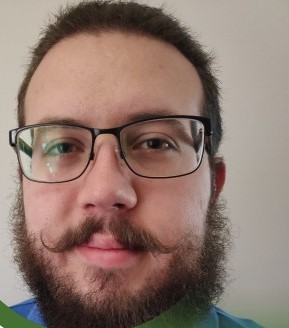
Previously at
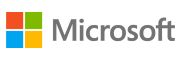
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git