How to create a Python class?
In Python, classes are the foundation for object-oriented programming. They provide a means of bundling data and methods operating on that data into a single unit, encapsulating behaviors and properties that define objects.
Creating a Class in Python:
- Basic Class Definition:
Defining a class in Python starts with the `class` keyword, followed by the class name and a colon. By convention, class names use CamelCase notation.
```python class MyClass: pass ```
- Initialization Method:
When an object of the class is created, you often want to set its initial state. This is done with the `__init__` method, which is called a constructor.
```python class MyClass: def __init__(self, attribute1, attribute2): self.attribute1 = attribute1 self.attribute2 = attribute2 ```
- Adding Methods:
Methods define the behaviors associated with the class. They operate on the object’s data and can be defined like regular functions, but always include `self` as their first parameter.
```python class MyClass: def __init__(self, attribute1, attribute2): self.attribute1 = attribute1 self.attribute2 = attribute2 def my_method(self): return self.attribute1 + self.attribute2 ```
- Creating an Instance:
Once the class is defined, you can instantiate objects (or instances) of that class. This is done by calling the class name as if it were a function, passing any required initial arguments.
```python my_instance = MyClass("Hello", "World!") ```
- Accessing Attributes and Methods:
You can access or modify the object’s attributes and call its methods using the dot (`.`) notation.
```python print(my_instance.attribute1) # Outputs: Hello print(my_instance.my_method()) # Outputs: HelloWorld! ```
Classes in Python provide a structured and efficient way to model real-world objects and behaviors. They encapsulate data and the methods that operate on that data, enabling developers to create robust and organized code that’s easy to maintain and extend. Familiarity with creating and using classes is crucial for anyone delving into Python’s object-oriented capabilities.
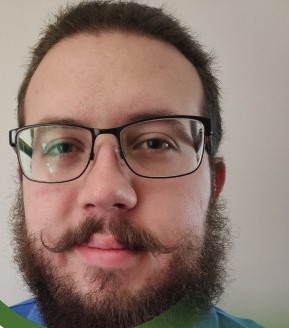
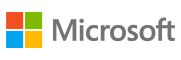