How to Create and Use Python Modules
Python is an extremely popular programming language due to its simplicity, versatility, and the breadth of its standard library. One feature of Python that facilitates the management and organization of large codebases is the concept of “modules”. These are separate Python script files that contain a collection of related functions and classes which can be “imported” and utilized in other Python scripts. In this blog post, we’ll delve into the steps for creating and using Python modules.
What is a Python Module?
A Python module is essentially a file containing Python code. The code can consist of functions, classes, or variables that are logically grouped together. The purpose of a module is to organize code in a manageable and efficient way, thereby promoting code reusability and maintainability.
How to Create a Python Module
Let’s dive into how you can create your own Python module. To start, you simply need to create a new Python file (a file with a `.py` extension). In this file, you can write any Python code, like functions and classes, that you wish to use in other scripts.
For example, let’s create a simple module for performing basic arithmetic operations. We will name it `arithmetic.py`.
# arithmetic.py def add(x, y): return x + y def subtract(x, y): return x - y def multiply(x, y): return x * y def divide(x, y): try: return x / y except ZeroDivisionError: return "Error: Division by zero is undefined"
In this `arithmetic.py` file, we’ve defined four functions: `add()`, `subtract()`, `multiply()`, and `divide()`. This file, when saved in the same directory as your other Python scripts, can now be used as a module.
Importing and Using a Python Module
Once you’ve created a module, you can use the functions and classes it contains in your other Python scripts through importing. The `import` statement in Python is used to load a module into your script’s memory.
Continuing with our arithmetic example, suppose we have another Python script called `main.py`. To use the `add` function from our `arithmetic` module in `main.py`, we’d need to import it like so:
# main.py import arithmetic result = arithmetic.add(5, 7) print(result) # Outputs: 12
Notice how we’ve used the module name followed by a dot (`.`) and the function name. This is known as dot notation and is a way to access the functions and classes in a module.
If you want to import only a specific function or class from a module, you can use the `from … import …` statement:
# main.py from arithmetic import add result = add(5, 7) print(result) # Outputs: 12
This way, you don’t need to use the module name before the function name.
Aliasing Modules and Functions
In Python, you can also provide an alias or a short name while importing a module or a function. This is particularly useful when dealing with modules with long names or if you wish to avoid naming conflicts. The `as` keyword is used to create an alias.
Here’s how you can alias our `arithmetic` module and `add` function:
# main.py import arithmetic as ar from arithmetic import subtract as sub add_result = ar.add(5, 7) print(add_result) # Outputs: 12 sub_result = sub(10, 3) print(sub_result) # Outputs <br />
: 7
Reloading a Python Module
Python modules are only loaded once per session by default. This means that if you make changes to a module after it has been imported, the changes won’t take effect in the current Python session.
However, you can use Python’s `importlib` module to reload a module. This forces Python to recompile the module and incorporate any changes made.
# main.py import arithmetic import importlib importlib.reload(arithmetic)
Remember, excessive use of `importlib.reload()` can make your code less readable and more error-prone, so use it judiciously.
The Python Standard Library
Besides creating your own modules, Python also comes with a vast standard library of pre-existing modules. These include modules for handling files, interacting with the operating system, managing datetime objects, and much more.
You can import and use these modules in the same way as your own. For example, the `math` module is a part of Python’s standard library:
# main.py import math print(math.sqrt(16)) # Outputs: 4.0
Conclusion
Python modules are instrumental in organizing and reusing code, making them indispensable for any Python programmer. Understanding how to create, use, and manage Python modules will allow you to write cleaner, more efficient, and better-organized code.
Remember, the key to mastering modules is practice. So, create your own modules, import them in your scripts, and explore the vast offerings of Python’s standard library. Happy coding!
Table of Contents
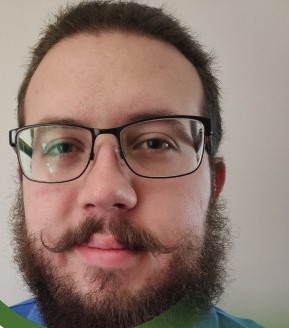
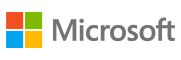