How to Use Python Functions for Data Cleaning
In the realm of data science and analysis, data cleaning is an indispensable process that lays the foundation for accurate and meaningful insights. Python, with its versatility and extensive library support, provides an excellent framework for data cleaning tasks. One of the key concepts that Python offers for organizing and simplifying data cleaning is the use of functions. In this guide, we’ll dive into the world of Python functions for data cleaning, exploring various techniques and best practices that can streamline your data preparation workflow.
Table of Contents
1. Introduction to Data Cleaning with Python Functions
1.1. Why Use Functions for Data Cleaning?
Functions are the building blocks of programming that encapsulate specific tasks and promote code modularity and reusability. When it comes to data cleaning, breaking down the process into smaller, focused functions can offer several advantages:
- Organization: Functions help you compartmentalize different cleaning tasks, making your codebase more organized and easier to maintain.
- Reusability: Once you’ve written a cleaning function for a specific task, you can reuse it across different datasets, reducing redundant coding efforts.
- Readability: Using descriptive function names and splitting tasks into smaller functions enhances code readability and makes it easier for others to understand your cleaning process.
- Scalability: As your data cleaning needs evolve, functions allow you to incrementally expand your cleaning toolkit without overhauling your entire codebase.
1.2. Benefits of Using Functions
When applying functions to data cleaning, you can reap numerous benefits:
- Efficiency: Functions enable you to automate repetitive tasks, leading to increased efficiency and reduced chances of human error.
- Consistency: By standardizing cleaning operations through functions, you ensure that the same steps are applied consistently to all data points.
- Testing and Debugging: Isolating cleaning tasks in functions makes it simpler to test individual components and identify and rectify errors.
- Collaboration: When working on a team, well-defined functions allow team members to collaborate more effectively, as each member can focus on different aspects of data cleaning.
2. Essential Python Functions for Data Cleaning
Let’s delve into some fundamental data cleaning tasks and explore how to implement them using Python functions.
2.1. Removing Duplicates – Ensuring Data Integrity
Duplicate entries can skew your analysis and lead to erroneous conclusions. Python’s pandas library offers a straightforward way to identify and remove duplicates:
python import pandas as pd def remove_duplicates(dataframe, subset=None): """ Remove duplicate rows from a DataFrame. Args: dataframe (pd.DataFrame): The input DataFrame. subset (list, optional): Columns to consider for identifying duplicates. Returns: pd.DataFrame: DataFrame with duplicate rows removed. """ cleaned_data = dataframe.drop_duplicates(subset=subset) return cleaned_data # Usage data = pd.read_csv('data.csv') cleaned_data = remove_duplicates(data, subset=['column1', 'column2'])
2.2. Handling Missing Values – Enhancing Data Completeness
Missing data can hinder accurate analysis. Python provides methods to handle missing values efficiently:
python def handle_missing_values(dataframe, strategy='mean'): """ Handle missing values in a DataFrame. Args: dataframe (pd.DataFrame): The input DataFrame. strategy (str): Strategy to fill missing values ('mean', 'median', 'forward_fill', 'backward_fill'). Returns: pd.DataFrame: DataFrame with missing values handled. """ if strategy == 'mean': filled_data = dataframe.fillna(dataframe.mean()) elif strategy == 'median': filled_data = dataframe.fillna(dataframe.median()) elif strategy == 'forward_fill': filled_data = dataframe.ffill() elif strategy == 'backward_fill': filled_data = dataframe.bfill() return filled_data # Usage data = pd.read_csv('data.csv') cleaned_data = handle_missing_values(data, strategy='mean')
2.3. String Cleaning and Standardization – Consistent Formatting
Inconsistent string formats can make data analysis challenging. Python’s string manipulation capabilities come in handy:
python def clean_strings(series): """ Clean and standardize strings in a Series. Args: series (pd.Series): The input Series with strings. Returns: pd.Series: Series with cleaned and standardized strings. """ cleaned_series = series.str.strip().str.lower() # Example: Strip leading/trailing spaces and convert to lowercase return cleaned_series # Usage data['name'] = clean_strings(data['name'])
2.4. Numeric Data Cleaning – Dealing with Outliers
Outliers can skew statistical analysis. Python provides tools to identify and handle outliers:
python def handle_outliers(series, method='z-score', threshold=2): """ Handle outliers in a numeric Series. Args: series (pd.Series): The input numeric Series. method (str): Outlier detection method ('z-score', 'IQR'). threshold (float): Threshold for identifying outliers. Returns: pd.Series: Series with outliers handled. """ if method == 'z-score': z_scores = (series - series.mean()) / series.std() cleaned_series = series[abs(z_scores) <= threshold] elif method == 'IQR': Q1 = series.quantile(0.25) Q3 = series.quantile(0.75) IQR = Q3 - Q1 lower_bound = Q1 - threshold * IQR upper_bound = Q3 + threshold * IQR cleaned_series = series[(series >= lower_bound) & (series <= upper_bound)] return cleaned_series # Usage data['age'] = handle_outliers(data['age'], method='z-score', threshold=2)
2.5. Datetime Cleaning – Handling Time-based Data
Dealing with datetime data involves parsing, formatting, and handling time zones. Python simplifies these tasks:
python def clean_datetime(series, format='%Y-%m-%d'): """ Clean and format datetime strings in a Series. Args: series (pd.Series): The input Series with datetime strings. format (str): Desired datetime format. Returns: pd.Series: Series with cleaned and formatted datetime objects. """ cleaned_series = pd.to_datetime(series, format=format, errors='coerce') return cleaned_series # Usage data['date'] = clean_datetime(data['date'], format='%d-%m-%Y')
2.6. Categorical Data Cleaning – Grouping and Aggregating
Cleaning categorical data often involves grouping and aggregating values. Python’s pandas library offers versatile tools for these tasks:
python def aggregate_categorical(dataframe, group_column, agg_column, aggregation='count'): """ Aggregate categorical data based on a grouping column. Args: dataframe (pd.DataFrame): The input DataFrame. group_column (str): Column for grouping data. agg_column (str): Column to perform aggregation on. aggregation (str): Aggregation function ('count', 'sum', 'mean', 'median'). Returns: pd.DataFrame: Aggregated DataFrame. """ if aggregation == 'count': aggregated_data = dataframe.groupby(group_column)[agg_column].count() elif aggregation == 'sum': aggregated_data = dataframe.groupby(group_column)[agg_column].sum() elif aggregation == 'mean': aggregated_data = dataframe.groupby(group_column)[agg_column].mean() elif aggregation == 'median': aggregated_data = dataframe.groupby(group_column)[agg_column].median() return aggregated_data.reset_index() # Usage grouped_data = aggregate_categorical(data, group_column='category', agg_column='sales', aggregation='sum')
3. Best Practices for Writing Data Cleaning Functions
To ensure your data cleaning functions are effective and maintainable, consider these best practices:
- Modularization: Break down the cleaning process into smaller functions that handle specific tasks. This enhances code organization and reusability.
- Parameterization: Design functions with flexible parameters to accommodate variations in cleaning strategies and data structures.
- Documentation: Provide clear and concise docstrings for each function, explaining its purpose, input parameters, and expected output.
- Error Handling: Implement proper error handling to gracefully manage unexpected situations, such as invalid inputs or failed operations.
4. Real-world Examples
Example 1: Cleaning Sales Data
Imagine you have a dataset containing sales data with duplicate entries and missing values. You can use the previously defined functions to clean the data effectively:
python import pandas as pd # Load data data = pd.read_csv('sales_data.csv') # Remove duplicates cleaned_data = remove_duplicates(data, subset=['customer_id', 'product_id']) # Handle missing values cleaned_data = handle_missing_values(cleaned_data, strategy='mean') # Aggregate sales by product category aggregated_data = aggregate_categorical(cleaned_data, group_column='category', agg_column='sales', aggregation='sum')
Example 2: Preprocessing Textual Data
Consider a scenario where you’re dealing with textual data that requires cleaning before natural language processing:
python # Load text data text_data = pd.read_csv('text_data.csv') # Clean and standardize text text_data['cleaned_text'] = clean_strings(text_data['raw_text']) # Tokenize and remove stopwords def preprocess_text(text): tokens = text.split() cleaned_tokens = [token for token in tokens if token not in stopwords] return ' '.join(cleaned_tokens) text_data['processed_text'] = text_data['cleaned_text'].apply(preprocess_text)
Conclusion
Data cleaning is a critical step in the data analysis process, and Python’s functions provide a powerful approach to tackle this task effectively. By modularizing cleaning tasks, handling duplicates, missing values, outliers, and standardizing data formats, you can prepare your data for meaningful insights. Remember to adhere to best practices, such as modularization, parameterization, documentation, and error handling, to create maintainable and robust data cleaning functions. Armed with the knowledge and techniques covered in this guide, you’re ready to embark on your data cleaning journey with Python functions, enhancing the quality and accuracy of your analytical endeavors.
Table of Contents
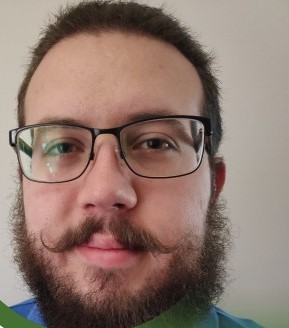
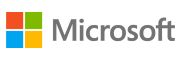