The Python Data Visualization Toolkit: Top 10 Libraries You Should Know
As data grows more complex, so does the need to effectively analyze and visualize it. Python, a popular programming language renowned for its readability and versatility, has a multitude of libraries that help data scientists visualize and comprehend data more effectively. The expertise of these Python tools is often best handled by professionals, hence why many businesses opt to hire Python developers. This blog post will introduce you to ten Python libraries for data visualization that both you and your hired Python developers should know.
1. Matplotlib
Matplotlib is the most widely used Python library for data visualization. It is a low-level library with a broad range of capabilities. You can create bar plots, histograms, scatter plots, and much more with just a few lines of code.
Consider the following example where we plot a simple line graph.
```python import matplotlib.pyplot as plt import numpy as np x = np.linspace(0, 10, 100) y = np.sin(x) plt.plot(x, y) plt.show() ```
This code produces a sine wave plot, demonstrating Matplotlib’s simplicity and efficiency.
2. Seaborn
Seaborn is built on top of Matplotlib and integrates well with pandas data structures. It provides a high-level interface for drawing attractive and informative statistical graphics. In addition to the basic functionality provided by Matplotlib, Seaborn also supports more complex visualization techniques like heatmap, pairplot, and violin plot.
```python import seaborn as sns import pandas as pd # Load the example iris dataset iris = sns.load_dataset("iris") # Create a pairplot sns.pairplot(iris, hue="species") plt.show() ```
The above code loads the iris dataset from seaborn and plots a pairplot that shows relationships between different features in the iris dataset.
3. Plotly
Plotly is a multi-platform data visualization library that allows interactive plots. Plotly’s graphs are visually attractive and present data in a detailed manner. These interactive plots are easily shareable and can be embedded in websites or applications.
```python import plotly.express as px df = px.data.iris() fig = px.scatter(df, x="sepal_width", y="sepal_length", color="species") fig.show() ```
The above code generates an interactive scatter plot for the iris dataset, where points are colored based on the species of the iris.
4. Bokeh
Similar to Plotly, Bokeh is another library that specializes in creating interactive visualizations. However, Bokeh puts an emphasis on server-side applications, which can be useful in more extensive data analysis projects.
```python from bokeh.plotting import figure, output_file, show output_file("line.html") p = figure(plot_width=400, plot_height=400) p.line([1, 2, 3, 4, 5], [6, 7, 2, 4, 5], line_width=2) show(p) ```
This code creates an HTML file with an interactive line plot.
5. ggplot
The ggplot library in Python is based on the ggplot2 package in R, which is renowned for its simplicity and flexibility. ggplot follows the Grammar of Graphics principles and allows users to create complex multi-layered graphics.
```python from ggplot import * p = ggplot(aes(x='date', y='beef'), data=meat) + geom_line() + stat_smooth(colour='blue', span=0.2) p ```
This code generates a smoothed line plot of beef consumption over time.
6. Altair
Altair is a declarative statistical visualization library based on Vega and Vega-Lite. Altair’s API is simple, friendly, and consistent and built on powerful and flexible underlying visualization specifications.
```python import altair as alt from vega_datasets import data iris = data.iris() alt.Chart(iris).mark_point().encode( x='petalLength', y='petalWidth', color='species' ) ```
The above code creates a point chart showing petal length against petal width, colored by species.
7. Pygal
Pygal is a dynamic SVG charting library. Pygal’s primary use case is creating SVGs or vector graphs. It is also interactive with tooltips, and the resulting charts are both minimal and beautifully styled.
```python import pygal bar_chart = pygal.Bar() bar_chart.add('Fibonacci', [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55]) bar_chart.render_to_file('bar_chart.svg') ```
This code creates a bar chart of the Fibonacci sequence and saves it as an SVG file.
8. Plotnine
Plotnine is another Python data visualization library based on ggplot2’s Grammar of Graphics. It provides a high-level interface for drawing informative and attractive statistical graphics.
```python from plotnine import ggplot, aes, geom_line from plotnine.data import economics df = economics (ggplot(df) + aes(x='date', y='pop') + geom_line() ) ```
This code generates a line plot of population over time from the economics dataset.
9. Folium
Folium builds on the data wrangling strengths of the Python ecosystem and the mapping strengths of the Leaflet.js library. It helps manipulate your data in Python and then visualize it on a Leaflet map via Folium.
```python import folium m = folium.Map(location=[45.5236, -122.6750]) m.save('map.html') ```
This code creates a map centered at given coordinates, and then it saves the map as an HTML file.
10. NetworkX
NetworkX is a Python package used for creating, manipulating, and studying the structure, dynamics, and functions of complex networks. While it’s not specifically a data visualization tool, it does provide functionalities to draw small networks and graphs.
```python import networkx as nx G = nx.Graph() G.add_edge('A', 'B') G.add_edge('B', 'C') nx.draw(G, with_labels=True) plt.show() ```
This code creates a graph with nodes A, B, and C and edges between A and B, and B and C.
Conclusion
These ten Python libraries each offer unique and robust capabilities for data visualization, and they often complement each other. For businesses looking to make the most of these tools, hire Python developers who can navigate these libraries with ease. Depending on your specific needs, you may find one library more suitable than the others. Exploring these libraries can provide powerful tools for understanding and presenting your data, especially when you have skilled Python developers at your service. Happy coding!
Table of Contents
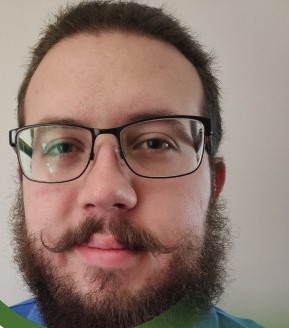
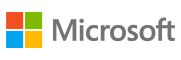