Python Tutorial: Understanding Dictionary and Set Data Types
Python, a popular programming language known for its simplicity and versatility, offers a wide range of built-in data types that cater to various programming needs. Among these, dictionaries and sets stand out as powerful data types that allow for efficient storage, retrieval, and manipulation of data. In this tutorial, we’ll delve into the world of dictionaries and sets, exploring their features, use cases, and providing code samples to solidify your understanding.
Table of Contents
1. Introduction to Dictionaries and Sets
1.1. What are Dictionaries and Sets?
Dictionaries are versatile data structures in Python that store collections of key-value pairs. Unlike lists or tuples, where elements are accessed by their position, dictionaries allow you to access elements using their associated keys. This feature makes dictionaries highly efficient for scenarios where fast data retrieval is crucial.
Sets, on the other hand, are collections of unique elements. They are particularly useful when you need to maintain a collection of items without duplicates, or when you want to perform operations like union, intersection, and difference on your data.
1.2. Why Use Dictionaries and Sets?
Dictionaries are indispensable when you need to associate data with specific labels or identifiers. For instance, in a student database, you can store student information with unique student IDs as keys and corresponding details as values. This approach enables rapid data retrieval without the need for looping through the entire dataset.
Sets, on the other hand, excel at handling scenarios that involve distinct elements. Whether it’s a list of tags associated with an article or a collection of email addresses, sets ensure that each item is unique, eliminating redundancies.
2. Dictionaries: Unleashing Key-Value Pairs
2.1. Creating Dictionaries
Creating dictionaries in Python is straightforward. You enclose key-value pairs in curly braces {}, with keys and values separated by colons :. Let’s create a simple dictionary representing a person’s details:
python person = { "name": "John Doe", "age": 30, "occupation": "Engineer" }
2.2. Accessing and Modifying Elements
Accessing elements in a dictionary involves using the associated key. For instance, to retrieve the person’s name:
python print(person["name"]) # Output: John Doe
If the key is not present in the dictionary, a KeyError will be raised. To avoid this, you can use the get() method:
python print(person.get("gender", "Unknown")) # Output: Unknown
To modify an existing value or add a new key-value pair:
python person["age"] = 31 # Modifying age person["gender"] = "Male" # Adding a new key-value pair
2.3. Iterating Through a Dictionary
You can iterate through a dictionary using loops. By default, a loop iterates through the keys:
python for key in person: print(key, person[key])
If you want to iterate through both keys and values simultaneously, use the items() method:
python for key, value in person.items(): print(key, value)
2.4. Dictionary Comprehensions
Similar to list comprehensions, dictionary comprehensions provide a concise way to create dictionaries. For instance, let’s create a dictionary of squares:
python squares = {x: x ** 2 for x in range(1, 6)} # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
3. Sets: Uniqueness and Set Operations
3.1. Creating Sets
Sets are created by enclosing elements in curly braces {}. Duplicate elements are automatically eliminated:
python tags = {"python", "tutorial", "python", "programming"} print(tags) # Output: {'python', 'tutorial', 'programming'}
3.2. Adding and Removing Elements
To add elements to a set, use the add() method:
python tags.add("coding") print(tags) # Output: {'python', 'tutorial', 'programming', 'coding'}
To remove elements, use the remove() method. If the element is not present, a KeyError will be raised. Alternatively, you can use discard() to avoid errors:
python tags.remove("tutorial") tags.discard("nonexistent_tag")
3.3. Set Operations: Union, Intersection, Difference
Sets support various operations that facilitate data manipulation. Consider two sets, set1 and set2:
python set1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} Union: set1 | set2 or set1.union(set2) Intersection: set1 & set2 or set1.intersection(set2) Difference: set1 - set2 or set1.difference(set2)
3.4. Set Comprehensions
Just like list and dictionary comprehensions, you can create sets using set comprehensions. Here’s an example that generates a set of squares:
python squares = {x ** 2 for x in range(1, 6)} # Output: {1, 4, 9, 16, 25}
4. Use Cases for Dictionaries and Sets
4.1. Dictionaries for Data Mapping
Dictionaries shine when you need to map one set of data to another. Consider a scenario where you’re building a translation app:
python translations = { "hello": "??????", "world": "????" } print(translations["hello"]) # Output: ??????
4.2. Sets for Unique Data and Membership Checks
Sets are ideal for maintaining collections of unique items and performing membership checks. Here’s an example of checking common interests between users:
python user1_interests = {"coding", "reading", "gaming"} user2_interests = {"reading", "traveling"} common_interests = user1_interests.intersection(user2_interests) print(common_interests) # Output: {'reading'}
5. Best Practices and Tips
5.1. Dictionary and Set Performance
Dictionaries offer O(1) average-case time complexity for data retrieval, making them incredibly fast. Sets also provide quick membership checks.
5.2. Handling Key Errors
When accessing dictionary elements, use the get() method to avoid KeyError exceptions. For sets, use discard() to avoid errors when removing elements.
5.3. Choosing Between Dictionaries and Lists
Use dictionaries when data retrieval by key is a priority. For lists, opt for sets when uniqueness is essential.
6. Code Samples and Examples
Example 1: Creating and Manipulating a Dictionary
python person = { "name": "Alice", "age": 25, "occupation": "Designer" } print(person["age"]) # Output: 25 person["occupation"] = "Artist" person["gender"] = "Female" print(person) # Output: {'name': 'Alice', 'age': 25, 'occupation': 'Artist', 'gender': 'Female'}
Example 2: Performing Set Operations
python set1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} union_result = set1.union(set2) intersection_result = set1.intersection(set2) difference_result = set1.difference(set2) print(union_result) # Output: {1, 2, 3, 4, 5, 6} print(intersection_result) # Output: {3, 4} print(difference_result) # Output: {1, 2}
Example 3: Applying Dictionary Comprehensions
python numbers = {1, 2, 3, 4, 5} squared_dict = {num: num ** 2 for num in numbers} print(squared_dict) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Example 4: Using Set Comprehensions
python numbers = {1, 2, 3, 4, 5} squared_set = {num ** 2 for num in numbers} print(squared_set) # Output: {1, 4, 9, 16, 25}
Conclusion
Dictionaries and sets are invaluable tools in Python’s data manipulation arsenal. Dictionaries enable efficient mapping of data, while sets ensure uniqueness and facilitate set operations. By mastering these data types, you’ll enhance your ability to tackle a wide range of programming challenges efficiently and effectively. Happy coding!
Table of Contents
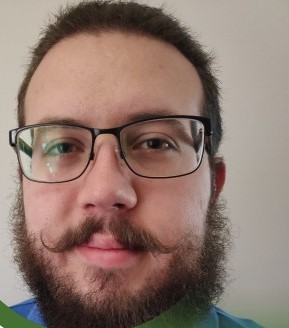
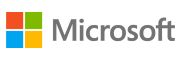