How to Use Python Functions for Email Automation
In today’s fast-paced world, automation has become a crucial aspect of increasing productivity and efficiency. Email automation, in particular, can save a significant amount of time by streamlining repetitive tasks. Python, being a versatile and popular programming language, offers a wide range of tools and libraries that can simplify email automation. In this blog, we will explore how to utilize Python functions to automate your email communication. From sending personalized messages to scheduling emails, you will discover how to harness the power of Python to supercharge your email workflow.
1. Why Use Python Functions for Email Automation?
Python’s popularity stems from its simplicity and readability. Functions are a fundamental building block of Python, allowing you to encapsulate code into reusable blocks. When it comes to email automation, functions can be incredibly handy, making it easier to organize and maintain your email-related tasks. Moreover, using functions enhances the readability of your code, making it more maintainable in the long run.
2. Setting Up the Environment
Before diving into email automation, you need to ensure you have Python installed on your system. You can download the latest version of Python from the official website (https://www.python.org/downloads/) and install it following the instructions for your specific operating system.
Once Python is set up, you’ll need to install the required libraries for email automation. We will be using the smtplib, email, and schedule libraries in this blog. To install these libraries, you can use the following pip commands:
python pip install smtplib pip install email pip install schedule
3. Sending Basic Emails
Using the smtplib Library:
The smtplib library in Python provides a simple way to send emails using the Simple Mail Transfer Protocol (SMTP). To send a basic email, you’ll need an SMTP server, the sender’s email address, and the recipient’s email address.
Here’s a code snippet demonstrating how to send a basic email:
python import smtplib def send_email(sender_email, sender_password, recipient_email, subject, body): try: server = smtplib.SMTP("smtp.gmail.com", 587) # Use the appropriate SMTP server and port server.starttls() server.login(sender_email, sender_password) message = f"Subject: {subject}\n\n{body}" server.sendmail(sender_email, recipient_email, message) server.quit() print("Email sent successfully!") except Exception as e: print(f"Error: {e}")
4. Sending Personalized Emails
Reading Email Templates from Files:
Sending personalized emails often involves using templates that contain variables to be replaced with specific values for each recipient. Storing these templates in separate files makes it easier to manage and modify them without changing the Python code.
Let’s assume you have a template file named “email_template.txt” with the following content:
css Dear {name}, Thank you for being a valued customer of our company. Best regards, The XYZ Team
Incorporating Variables in Email Content:
To send personalized emails, we can modify our send_email function to read the template from a file and replace the variables with the appropriate values for each recipient.
python def send_personalized_email(sender_email, sender_password, recipient_email, subject, template_file, replacements): try: with open(template_file, "r") as file: template = file.read() for key, value in replacements.items(): template = template.replace("{" + key + "}", value) send_email(sender_email, sender_password, recipient_email, subject, template) except Exception as e: print(f"Error: {e}")
Here, the replacements parameter is a dictionary containing variable names as keys and the corresponding values for each recipient. For example, if you want to send personalized emails to John and Jane, you would call the function like this:
python template_file = "email_template.txt" john_replacements = {"name": "John Doe"} jane_replacements = {"name": "Jane Smith"} send_personalized_email(sender_email, sender_password, "john@example.com", "Personalized Greetings", template_file, john_replacements) send_personalized_email(sender_email, sender_password, "jane@example.com", "Personalized Greetings", template_file, jane_replacements)
5. Bulk Email Sending
Reading Email Addresses from a CSV File:
In some scenarios, you might need to send emails to a large number of recipients. Instead of hardcoding each recipient’s email address, you can store them in a CSV file and read them programmatically.
Let’s assume you have a CSV file named “recipients.csv” with the following content:
graphql email john@example.com jane@example.com jim@example.com …
Automating the Sending Process:
To automate bulk email sending, we can enhance our function to read email addresses from the CSV file and send personalized emails to each recipient.
python import csv def send_bulk_emails(sender_email, sender_password, subject, template_file, csv_file): try: with open(csv_file, "r") as file: reader = csv.DictReader(file) for row in reader: recipient_email = row["email"] send_personalized_email(sender_email, sender_password, recipient_email, subject, template_file, replacements={}) except Exception as e: print(f"Error: {e}")
Using this function, you can send personalized emails to all recipients listed in the CSV file with just one function call:
python template_file = "email_template.txt" csv_file = "recipients.csv" send_bulk_emails(sender_email, sender_password, "Bulk Email Greetings", template_file, csv_file)
6. Scheduling Emails
Utilizing the schedule Library:
Python’s schedule library allows you to schedule tasks at specific intervals. We can leverage this library to automate the process of sending emails at designated times.
To use the schedule library, install it using pip:
python pip install schedule
Automating Email Delivery at Specific Times:
Here’s a function that schedules the bulk email sending task to be executed at a specific time:
python import schedule import time def schedule_bulk_emails(sender_email, sender_password, subject, template_file, csv_file, send_time): try: schedule.every().day.at(send_time).do(send_bulk_emails, sender_email, sender_password, subject, template_file, csv_file) while True: schedule.run_pending() time.sleep(1) except Exception as e: print(f"Error: {e}")
To schedule the bulk email sending to occur every day at 9 AM, you can use the following code:
python template_file = "email_template.txt" csv_file = "recipients.csv" schedule_bulk_emails(sender_email, sender_password, "Scheduled Greetings", template_file, csv_file, "09:00")
7. Email Validation
Checking Email Addresses for Validity:
Before sending emails, it’s essential to validate the recipient email addresses to ensure successful delivery. Python provides several libraries for email address validation, such as the validate_email library.
Install the validate_email library using pip:
python pip install validate_email
Handling Invalid Addresses:
Here’s a function that incorporates email validation and handles any invalid email addresses in the CSV file:
python from validate_email import validate_email def send_valid_bulk_emails(sender_email, sender_password, subject, template_file, csv_file): try: with open(csv_file, "r") as file: reader = csv.DictReader(file) for row in reader: recipient_email = row["email"] if validate_email(recipient_email): send_personalized_email(sender_email, sender_password, recipient_email, subject, template_file, replacements={}) else: print(f"Invalid email address: {recipient_email}") except Exception as e: print(f"Error: {e}")
Using this function, you can automatically skip invalid email addresses and send personalized emails only to valid recipients:
python template_file = "email_template.txt" csv_file = "recipients.csv" send_valid_bulk_emails(sender_email, sender_password, "Valid Bulk Greetings", template_file, csv_file)
Conclusion:
Python’s versatility and ease of use make it an excellent choice for automating various tasks, including email communication. In this blog, we explored how to leverage Python functions for email automation, from sending basic emails to scheduling bulk email delivery. By combining Python with its powerful libraries, you can streamline your email workflow, save time, and enhance productivity. Whether you need to send personalized messages or automate bulk email delivery, Python’s robust features empower you to achieve seamless email automation effortlessly. Happy coding!
Table of Contents
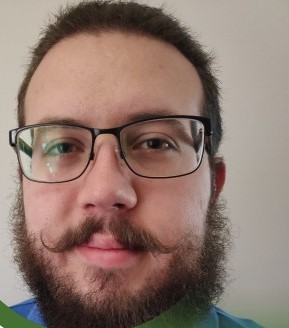
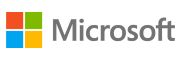