How to handle exceptions in Python?
Exception handling is a crucial aspect of writing robust code in Python. Exceptions are unforeseen errors that arise during program execution, and if not handled, can cause your program to crash. Python provides a powerful system to capture and manage these exceptions using the `try`, `except`, `else`, and `finally` blocks.
Basic Exception Handling:
The core idea is to wrap potentially problematic code inside a `try` block and then specify how to handle the error(s) in the subsequent `except` block.
```python try: result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero!") ```
In the above example, attempting to divide by zero raises a `ZeroDivisionError`. The `except` block captures this exception and prints an error message.
Handling Multiple Exceptions:
You can handle multiple exceptions by specifying multiple `except` blocks.
```python try: # some code that might raise exceptions except (TypeError, ValueError) as e: print(f"Caught an error: {e}") ```
Using `else` and `finally`:
– The `else` block is executed if the `try` block doesn’t raise any exceptions. It’s often used to specify code that must run if there were no errors.
– The `finally` block always runs, whether an exception occurred or not. It’s typically used for cleanup operations, like closing files or network connections.
```python try: # some code except SomeException: # handle exception else: # runs if there's no exception in the try block finally: # always runs, regardless of exceptions ```
Raising Exceptions:
You can raise exceptions using the `raise` keyword, either re-throwing caught exceptions or generating new ones based on custom conditions.
```python if some_condition: raise ValueError("A custom error message") ```
Custom Exceptions:
For more specialized error handling, Python allows you to define custom exception classes. These classes typically derive from the base `Exception` class or its subclasses.
```python class CustomError(Exception): pass ```
Handling exceptions in Python ensures the graceful management of unexpected errors, enhancing the reliability and user experience of your software. By understanding and utilizing the provided constructs, you can bulletproof your code against unforeseen issues.
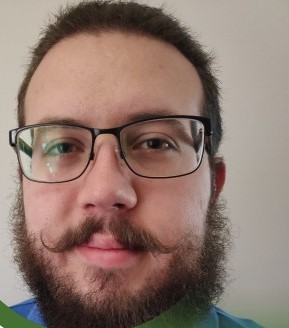
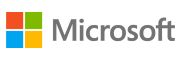