How to execute shell commands from within Python?
Executing shell commands directly from a Python script can be incredibly useful for automating tasks, system administration, or integrating different software components. Here’s a concise overview on how to run shell commands from within Python:
- Using the `os.system()` Method:
The `os` module in the Python Standard Library provides the `system()` function, which allows you to run shell commands directly from your script. This method returns the command’s exit code and allows for easy command execution. However, it doesn’t give much control over input/output redirection or capturing command output.
- Using the `subprocess` Module:
For more robust and flexible command execution, the `subprocess` module is the preferred choice. This module provides a higher-level interface to spawn subprocesses and interact with their input/output/error pipes and obtain their return codes.
– `subprocess.run()`: This is a simple way to run a command in a subprocess. It returns a `CompletedProcess` object once the command completes, from which you can extract the return code, stdout, and stderr.
– `subprocess.Popen()`: This is a more flexible interface, allowing for fine-grained control over input and output streams, waiting for processes, and more.
- Capturing Command Output:
If you want to capture the output of a command for further processing in your script, the `subprocess` module is again the tool of choice. By using the `run()` function with the `capture_output=True` argument or by setting the `stdout` and `stderr` arguments of `Popen()`, you can capture the output and error streams of the command.
- Safety Considerations:
It’s essential to be cautious when executing shell commands, especially if incorporating user input. There’s a risk of command injection attacks, where malicious input can cause unintended command execution. Always validate and sanitize input and consider using shell=False to avoid shell-specific security vulnerabilities.
While Python offers multiple ways to execute shell commands, the `subprocess` module is the most versatile and recommended approach for modern applications. Its flexibility allows for direct command execution, capturing output, and safe interaction with subprocesses. Regardless of the method chosen, always remain cautious of potential security implications and sanitize any inputs that might be used in command execution.
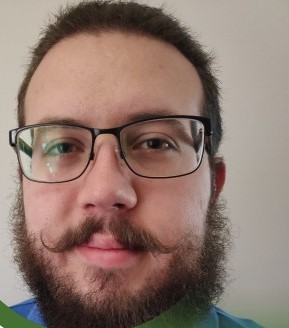
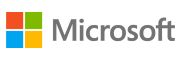