Working with Files and Directories in Python
Welcome to our detailed tutorial on “Python Tutorial: Working with Files and Directories”. This guide will help you understand how to efficiently manage and manipulate files and directories using Python. From reading and writing files to creating, renaming, and deleting directories, we’ll cover a wide range of operations essential for any Python programmer. We’ll also delve into error handling and path manipulations. By the end of this tutorial, you’ll be well-equipped to handle file and directory operations in your Python projects.
File Operations in Python
Opening a File
In Python, the built-in `open()` function is used to open a file. The function takes two arguments: the filename and the mode of operation.
file = open('myfile.txt', 'r')
The modes can be read (`’r’`), write (`’w’`), append (`’a’`), and more. The `’r’` mode is the default mode if not provided.
Reading from a File
To read the contents of a file, use the `read()` method. Here’s how:
file = open('myfile.txt', 'r') content = file.read() print(content) file.close()
The `read()` method returns the contents of the file as a string. Remember to close the file using the `close()` method after you’re done.
To read lines into a list, use `readlines()`:
file = open('myfile.txt', 'r') lines = file.readlines() print(lines) file.close()
Writing to a File
To write to a file, open it in write or append mode, then use the `write()` method.
file = open('myfile.txt', 'w') file.write('Hello, Python!') file.close()
Closing a File
Closing a file releases resources associated with it. It is a good practice to always close your files after working with them.
file = open('myfile.txt', 'r') # do stuff... file.close()
To ensure files are always closed properly, you can use the `with` statement which automatically closes the file when the nested block of code is done.
with open('myfile.txt', 'r') as file: print(file.read())
Working with Directories
Python’s built-in `os` module allows us to interact with the underlying operating system, including working with directories (folders).
Getting the Current Directory
To get the path of the current directory, use `os.getcwd()`:
import os print(os.getcwd())
Changing the Current Directory
To change the current directory, use `os.chdir()`:
os.chdir('/path/to/directory')
Listing Files in a Directory
To list all files and directories in the current directory, use `os.listdir()`:
print(os.listdir())
Creating a Directory
To create a directory, use `os.mkdir()`:
os.mkdir('new_directory')
Deleting a Directory
To delete a directory, use `os.rmdir()`:
os.rmdir('directory_to_delete')
Please note that `os.rmdir()` only removes empty directories. To remove a directory and all its contents, use `shutil.rmtree()`:
import shutil shutil.rmtree('directory_to_delete')
Handling File and Directory Paths
Python’s `os.path` module contains methods for manipulating file and directory paths.
Joining Paths
To join two or more pathname components, use `os.path.join()`:
print(os.path.join('/path/to/', 'file.txt'))
This method takes care of any necessary syntax details such as the addition of slashes.
Checking Path Validity
To check whether a path exists, use `os.path.exists()`:
print(os.path.exists('/path/to/file.txt'))
Splitting Path Names
To split a pathname into a pair (head, tail), use `os.path.split()`. The tail part is the last pathname component, and the head part is everything leading up to that.
print(os.path.split('/path/to/file.txt'))
Error Handling
When working with files and directories, errors are common. Python’s built-in exception handling can be used to elegantly handle these.
try: file = open('non_existent_file.txt', 'r') except FileNotFoundError: print('File not found.')
Conclusion
This tutorial explored the basic operations for working with files and directories in Python, including opening and closing files, reading and writing data, navigating directories, and manipulating file paths. The built-in `os` module provides a range of useful functions for interacting with the underlying operating system. Remember to handle potential errors to create robust and reliable code.
Practice these techniques and you’ll find manipulating files and directories in Python second nature in no time! Happy coding!
Table of Contents
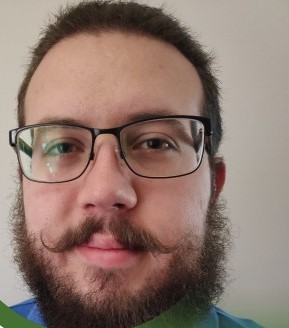
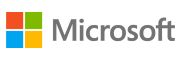