Empowering Your Data Analysis Skills with Python Functions
Python is a popular and versatile programming language used across many spheres of technology, including data analysis. Its versatility and wide range of functionalities make it a top choice for companies looking to hire Python developers. Python offers various built-in functions and libraries such as Pandas, NumPy, and Matplotlib, which are incredibly useful for analyzing and visualizing data. This blog post will demonstrate how to use Python functions effectively for data analysis, including examples. It can serve as a useful guide not just for data analysts, but also for businesses seeking to hire Python developers to gain insights from their data.
Why Python for Data Analysis?
Python is a great language for data analysis for several reasons:
- Readability: Python’s clear syntax makes it easy to read, write, and understand.
- Extensive Libraries: Python has a robust ecosystem of libraries (e.g., Pandas, NumPy, Matplotlib, Seaborn) specifically designed for data analysis and visualization.
- Integration: Python easily integrates with other languages and platforms, making it very versatile.
- Community Support: Python has a large and growing community of users who are always contributing to its development, providing support, and creating new libraries and tools.
Let’s jump into understanding some of the most frequently used Python functions in data analysis.
Basic Python Functions
Let’s start with some basic Python functions useful in data analysis.
The print() Function
The `print()` function is one of the simplest yet most frequently used Python functions. It outputs to the console whatever you place inside the parentheses.
```python print("Hello, World!") ``` Output: ``` Hello, World! ```
The len() Function
The `len()` function is used to find the number of elements in a list, characters in a string, keys in a dictionary, etc.
```python my_list = [1, 2, 3, 4, 5] print(len(my_list)) ``` Output: ``` 5 ```
The type() Function
The `type()` function returns the datatype of the variable or value you pass into it.
```python print(type(10)) print(type("Hello, World!")) print(type([1, 2, 3])) ``` Output: ``` <class 'int'> <class 'str'> <class 'list'> ```
Pandas for Data Analysis
Pandas is a high-level data manipulation tool developed by Wes McKinney. It’s built on the Numpy package and its key data structure is the DataFrame.
Importing Data with Pandas
To import data, we use the `read_csv()` function in pandas. The file path is passed as a parameter to the function.
```python import pandas as pd data = pd.read_csv('filepath/filename.csv') ```
Understanding the Data
- head(): This function returns the first n rows for the object based on position. By default, it returns the first 5 rows.
```python data.head() ```
- info(): This function returns a concise summary of a DataFrame, including the number of non-null entries in each column.
```python data.info() ```
Cleaning the Data
Data cleaning can involve multiple steps. Let’s look at two of them:
- dropna(): This function allows you to drop all (or some) rows that have missing values.
```python cleaned_data = data.dropna() ```
- replace(): This function replaces a set of values with some other set of values.
```python data.replace(to_replace ="Old value", value ="New value") ```
NumPy for Data Analysis
NumPy, which stands for Numerical Python, is a library consisting of multidimensional array objects and a collection of routines for processing of arrays.
Creating an Array
```python import numpy as np a = np.array([1, 2, 3]) print(a) ``` Output: ``` [1 2 3] ```
Basic Array Operations
Operations such as addition, subtraction, multiplication, and division can be performed on NumPy arrays, as can many mathematical functions, like `sum()`, `mean()`, `max()`, and `min()`.
```python b = np.array([4, 5, 6]) # Addition print(a + b) # Subtraction print(a - b) # Multiplication print(a * b) # Division print(a / b) ```
Matplotlib for Data Visualization
Matplotlib is a Python 2D plotting library that enables you to produce figures and charts, both in a screen or hardcopy.
```python import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y = [10, 24, 36, 40, 5] # Create the plot plt.plot(x, y) # Show the plot plt.show() ```
Conclusion
Python provides a rich ecosystem for performing data analysis, which is why many companies opt to hire Python developers for their projects. While this blog post provided a starting point, there are many other functionalities and libraries to explore. As a data analyst or a Python developer, mastering these functions and learning to use them efficiently can give you a powerful toolset for uncovering insights within your data.
In a data-driven world, the demand to hire Python developers is on the rise due to the versatility and readability of the language. Whether you’re looking to enhance your own skill set or aiming to join a team as a Python developer, the best way to improve is through practice. So, get your hands dirty with some data and start analyzing!
Table of Contents
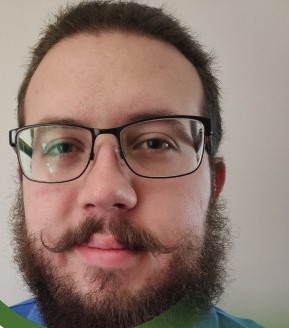
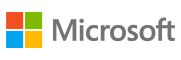