How to Use Python Functions for Web Scraping
In today’s digital age, the ability to access, organize, and analyze online data is more important than ever. One of the most efficient ways to gather data from the internet is through web scraping. Python, with its rich ecosystem of libraries and tools, makes the process of web scraping relatively straightforward.
Web scraping is a technique used to extract large amounts of data from websites. The data on the websites are unstructured, and web scraping enables us to convert that data into a structured form. In this post, we’ll explore how to use Python functions for web scraping.
Getting Started
First, let’s ensure we have the required libraries installed:
pip install requests pip install BeautifulSoup4
Requests allow us to send HTTP requests to get the HTML content of a webpage. BeautifulSoup is a Python library used for parsing HTML and XML documents and navigating, searching, and modifying the parse tree.
Basic Web Scraping with Python
Web scraping with Python generally involves these steps:
- Sending an HTTP request to the URL of the webpage you want to access. The server responds to the request by returning the HTML content of the webpage.
- Once you have accessed the HTML content, you are left with the task of parsing the data. Since most of the HTML data is nested, you cannot extract data simply through string processing. One needs a parser which can create a nested/tree structure of the HTML data. There are several HTML parser libraries available but the most advanced one is HTML5lib.
- Now that we have accessed the HTML content, we need to parse it to get meaningful data. This is where BeautifulSoup comes into play.
- After you have parsed and extracted structured data from the HTML content, you might want to store it. The choice of storage depends on your needs. The most common storage formats are CSV, Excel, and databases like MySQL.
Writing Your First Python Web Scraper
Let’s create a simple Python scraper that fetches and prints out the title from a webpage.
import requests from bs4 import BeautifulSoup def get_page_title(url): # Send a request to the website r = requests.get(url) # Get the content of the request content = r.text # Create a BeautifulSoup object and specify the parser soup = BeautifulSoup(content, 'html.parser') # Get the webpage title title = soup.title.string # Print the webpage title print(title)
You can use the function above like so:
get_page_title('https://www.example.com')
Advanced Web Scraping
For more advanced data extraction, you’ll need to learn about different HTML elements and how they can be accessed.
Say we want to extract all the links on a webpage. This is how you might do it:
def get_all_links(url): # Send a request to the website r = requests.get(url) # Get the content of the request content = r.text # Create a BeautifulSoup object and specify the parser soup = BeautifulSoup(content, 'html.parser') # Find all 'a' tags (which define hyperlinks): <a href="www.example.com">Link text</a> links = soup.find_all('a') # Loop through all found 'a' tags for link in links: # Get the link's URL (href attribute) and its text href = link.get('href') text = link.string print(f'URL: {href}, Text: {text}')
This function will print out all the URLs and their link texts on a webpage.
Web Scraping with Error Handling
When you’re scraping the web, you’ll encounter many different scenarios. For example, a page might not exist, or the server might not respond. To handle these scenarios, you can include error handling in your web scraping functions.
Here’s an example of a web scraping function with basic error handling:
def get_page_title(url): try: # Send a request to the website r = requests.get(url) # If the request was successful, no Exception will be raised except Exception as e: print(f"There was an error: {e}") return None # Get the content of the request content = r.text # Create a BeautifulSoup object and specify the parser soup = BeautifulSoup(content, 'html.parser') # Get the webpage title title = soup.title.string return title
This function will catch and print out any errors that happen when sending the request.
Conclusion
In this post, we’ve gone over the basics of web scraping with Python, using the requests and BeautifulSoup libraries. We’ve learned how to send a request to a website, parse the HTML content of a webpage, extract data, and handle basic errors.
Web scraping is a powerful tool to have in your data analysis arsenal. However, it’s important to use it responsibly to respect the privacy of others and comply with the terms of service of the website you are scraping.
Remember, Python is an excellent language for web scraping due to its ease of learning and the rich ecosystem of data science libraries. With Python, you can handle vast amounts of data from the web, and turn unstructured data into structured data that’s ready for analysis.
Happy scraping!
Table of Contents
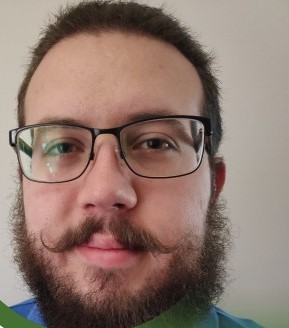
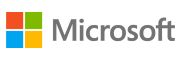