How to Use Python Functions to Connect to APIs
In today’s data-driven world, connecting to Application Programming Interfaces (APIs) is a crucial aspect of software development. APIs serve as bridges between different software systems, allowing them to communicate and share data seamlessly. Python, with its versatility and extensive libraries, makes it an excellent choice for API integration. In this blog, we will dive deep into the world of Python functions and explore how they can be utilized to connect to APIs, fetch data, and streamline your applications.
1. Understanding APIs and Python Functions:
What are APIs?
APIs (Application Programming Interfaces) are sets of rules and protocols that allow different software applications to interact and exchange data. They define how requests and responses should be structured, enabling seamless communication between diverse systems. APIs are commonly used by developers to access the functionality of web services, libraries, and other software components.
What are Python Functions?
In Python, functions are blocks of reusable code that perform a specific task. They help in organizing and modularizing code, making it more readable and maintainable. Python functions take inputs (arguments) and return outputs (return values) based on the logic defined within them. Functions in Python can be defined using the def keyword and can be called from other parts of the code.
2. Making API Requests with Python:
Installing Required Libraries:
Before we start making API requests in Python, we need to install the requests library, which simplifies the process of sending HTTP requests and handling responses. You can install it using pip:
python pip install requests
Sending GET Requests:
To retrieve data from an API, we usually use HTTP GET requests. Let’s see an example of how to make a GET request using Python:
python import requests def fetch_data_from_api(url): response = requests.get(url) return response # Example usage: api_url = "https://api.example.com/data" data_response = fetch_data_from_api(api_url) print(data_response.status_code) # Print the status code (200 for success) print(data_response.json()) # Print the JSON response data
Handling Responses:
The response received from the API can be processed further. In the example above, we used .json() to convert the response data to a Python dictionary when the API returned data in JSON format. Depending on the API, the response may be in other formats like XML or HTML, and you’ll need to handle it accordingly.
3. Authenticating with APIs:
API Keys and Authentication Methods:
Many APIs require authentication to control access and identify users. One common method of authentication is using an API key, a unique identifier provided by the API provider. Other authentication methods include OAuth tokens, username/password, or client certificates.
Incorporating Authentication into Requests:
Let’s see how to include an API key in the request headers for authentication:
python import requests def fetch_data_with_auth(api_url, api_key): headers = {"Authorization": f"Bearer {api_key}"} response = requests.get(api_url, headers=headers) return response # Example usage: api_url = "https://api.example.com/data" api_key = "your_api_key_here" data_response = fetch_data_with_auth(api_url, api_key) print(data_response.json())
4. Query Parameters and Endpoints:
Customizing API Requests:
APIs often accept query parameters to customize the data returned. For example, you might want to specify a date range, sorting options, or filters. These parameters are typically included in the URL of the request.
Handling Query Parameters:
Let’s see how to add query parameters to our API request:
python import requests def fetch_data_with_params(api_url, params): response = requests.get(api_url, params=params) return response # Example usage: api_url = "https://api.example.com/data" query_params = {"date": "2023-07-01", "category": "weather"} data_response = fetch_data_with_params(api_url, query_params) print(data_response.json())
5. Handling API Responses:
Parsing JSON Data:
Most APIs return data in JSON format, which Python can easily parse into dictionaries or lists using the json module. Let’s see an example of parsing JSON data from an API response:
python import requests def fetch_data_from_api(url): response = requests.get(url) if response.status_code == 200: data = response.json() return data else: return None # Example usage: api_url = "https://api.example.com/data" data = fetch_data_from_api(api_url) if data: print(data["result"]) else: print("Failed to fetch data from the API.")
Error Handling:
API requests can sometimes fail due to various reasons like network issues or incorrect parameters. Proper error handling is essential to make our code more robust. We can use Python’s try-except block to handle exceptions gracefully:
python import requests def fetch_data_from_api(url): try: response = requests.get(url) response.raise_for_status() # Raise an exception for 4xx or 5xx status codes data = response.json() return data except requests.exceptions.HTTPError as http_err: print(f"HTTP error occurred: {http_err}") except requests.exceptions.RequestException as req_err: print(f"Request error occurred: {req_err}") except Exception as err: print(f"Error occurred: {err}") return None # Example usage: api_url = "https://api.example.com/data" data = fetch_data_from_api(api_url) if data: print(data["result"]) else: print("Failed to fetch data from the API.")
6. Organizing Code with Functions:
Benefits of Using Functions:
Using functions helps in breaking down complex tasks into smaller, manageable pieces. It promotes reusability, readability, and maintainability of the code. Functions allow you to encapsulate functionality, making it easier to test and debug specific parts of your application.
Structuring Your API Integration Code:
When integrating APIs into your projects, it’s essential to structure your code to keep it organized and efficient. Here’s an example of how you can structure your API integration code:
python import requests def fetch_data_from_api(url, params=None, headers=None): response = requests.get(url, params=params, headers=headers) return response def process_data(data): # Process the data returned by the API # ... def main(): api_url = "https://api.example.com/data" query_params = {"date": "2023-07-01", "category": "weather"} api_key = "your_api_key_here" headers = {"Authorization": f"Bearer {api_key}"} data_response = fetch_data_from_api(api_url, params=query_params, headers=headers) if data_response.status_code == 200: data = data_response.json() processed_data = process_data(data) # Do something with processed_data else: print(f"Failed to fetch data from the API. Status code: {data_response.status_code}") if __name__ == "__main__": main()
7. Case Study: Weather Data Retrieval:
Setting up the Weather API:
For this case study, let’s use a simple weather API that provides current weather data for cities. To get started, sign up for a free API key from the provider and ensure you have the requests library installed.
Writing Functions for Weather Data:
We’ll create functions to interact with the weather API, fetch data, and display the results:
python import requests def fetch_weather_data(api_key, city): api_url = f"https://api.exampleweather.com/weather" params = {"q": city, "appid": api_key} response = requests.get(api_url, params=params) return response def display_weather_data(data): if data["cod"] == "200": print(f"Weather in {data['name']}:") print(f"Description: {data['weather'][0]['description']}") print(f"Temperature: {data['main']['temp']}°C") else: print(f"Failed to fetch weather data. Error code: {data['cod']}") def main(): api_key = "your_weather_api_key_here" city = "New York" weather_response = fetch_weather_data(api_key, city) if weather_response.status_code == 200: weather_data = weather_response.json() display_weather_data(weather_data) else: print(f"Failed to fetch weather data. Status code: {weather_response.status_code}") if __name__ == "__main__": main()
8. Best Practices for API Integration:
Caching Responses:
To reduce unnecessary API calls and improve performance, consider implementing caching mechanisms. Cache the API responses locally, and if the same request is made again, retrieve the data from the cache instead of making a fresh API call.
Rate Limiting and Throttling:
Respect API rate limits to avoid overloading the API server. APIs often have rate-limiting policies that restrict the number of requests you can make within a specific time window. Implement throttling to control the request rate and prevent being blocked by the API provider.
Exception Handling:
As we saw earlier, proper exception handling is crucial when working with APIs. It helps in gracefully handling errors and prevents the application from crashing due to unexpected issues.
Conclusion:
Python provides a powerful set of tools to connect and interact with APIs efficiently. With the help of Python functions, you can modularize your code, making it easier to maintain and scale. This blog covered the fundamentals of API integration, including authentication, query parameters, error handling, and best practices. Armed with this knowledge, you can now confidently incorporate various APIs into your Python projects, enriching them with real-time data and functionalities. Happy coding!
Table of Contents
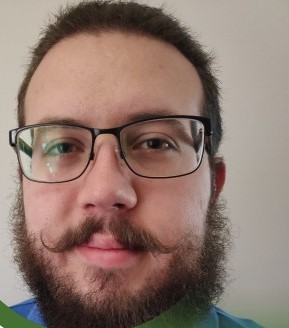
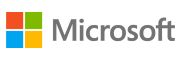