How to Use Python Functions to Create Interactive Charts
In today’s data-driven world, presenting information visually is essential for better understanding and analysis. Charts and graphs are powerful tools for visualizing data patterns, trends, and insights. Python, with its extensive libraries, offers fantastic capabilities for data visualization, enabling developers to create interactive charts with ease.
In this blog post, we’ll explore how to use Python functions to build interactive charts. We’ll focus on two popular libraries for data visualization: Matplotlib and Plotly. Whether you’re a beginner or an experienced Python developer, this guide will walk you through the steps to create visually appealing charts that engage your audience.
Introduction to Python
Python is a versatile programming language with numerous libraries that cater to various data manipulation and visualization needs. Among these, Matplotlib and Plotly stand out as popular choices for data visualization.
Matplotlib is a robust, flexible, and widely-used library that allows users to create static, high-quality charts. On the other hand, Plotly is a powerful library known for its interactive capabilities, enabling users to build dynamic and engaging visualizations.
Getting Started: Installing Required Libraries
Before we delve into creating interactive charts, make sure you have Python installed on your system. You can download the latest version of Python from the official website (https://www.python.org/downloads/).
To install the necessary libraries, open your terminal or command prompt and use the following commands:
python pip install matplotlib pip install plotly
With the required libraries installed, we are ready to dive into chart creation.
Creating Static Charts with Matplotlib
Matplotlib is a fantastic library for generating static charts and plots. It provides a wide range of customization options to design visually appealing visuals. Let’s explore how to create three common static charts: Line charts, Bar charts, and Pie charts.
Line Charts
Line charts are ideal for visualizing data trends over time or any ordered category. For instance, let’s plot a simple line chart to visualize the monthly revenue of a company for the last year:
python import matplotlib.pyplot as plt months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] revenue = [15000, 18000, 12000, 17000, 20000, 22000, 19000, 21000, 24000, 23000, 25000, 28000] plt.plot(months, revenue, marker='o') plt.xlabel('Months') plt.ylabel('Revenue ($)') plt.title('Monthly Revenue in the Last Year') plt.grid(True) plt.show()
In the above code snippet, we use the plt.plot() function to create a line chart. We customize the appearance using various functions like plt.xlabel(), plt.ylabel(), and plt.title() to add labels and a title to the chart. The plt.grid(True) line adds a grid to the chart, improving readability.
Bar Charts
Bar charts are useful for comparing categorical data or showing data distribution across different groups. Let’s create a simple bar chart to visualize the sales of different products:
python import matplotlib.pyplot as plt products = ['Product A', 'Product B', 'Product C', 'Product D'] sales = [120, 80, 150, 200] plt.bar(products, sales) plt.xlabel('Products') plt.ylabel('Sales') plt.title('Product Sales') plt.show()
In this example, we use the plt.bar() function to create a bar chart. We specify the products as the x-axis and the corresponding sales as the y-axis. The chart’s title and axes labels are added with the plt.xlabel(), plt.ylabel(), and plt.title() functions.
Pie Charts
Pie charts are suitable for displaying parts of a whole and are often used to represent percentages. Let’s visualize the percentage distribution of market shares for a company:
python import matplotlib.pyplot as plt markets = ['Market A', 'Market B', 'Market C', 'Market D'] market_shares = [30, 25, 15, 30] plt.pie(market_shares, labels=markets, autopct='%1.1f%%') plt.title('Market Share Distribution') plt.axis('equal') plt.show()
In this code snippet, we use the plt.pie() function to create a pie chart. The labels parameter assigns names to each section of the pie, and autopct specifies the format for displaying the percentages. The plt.axis(‘equal’) line ensures that the pie chart appears as a circle.
Enhancing Charts with Interactivity using Plotly
While Matplotlib allows us to create static charts, Plotly takes it a step further by enabling us to build interactive charts. These charts can be customized, and users can interact with them, gaining deeper insights into the data. Let’s dive into creating interactive versions of the line chart, bar chart, and pie chart using Plotly.
Scatter Plots
Scatter plots are excellent for visualizing relationships between two numerical variables. Let’s create an interactive scatter plot to represent the correlation between the hours spent studying and exam scores for a group of students:
python import plotly.express as px students = ['Alice', 'Bob', 'Charlie', 'David', 'Eve'] study_hours = [5, 3, 6, 4, 7] exam_scores = [85, 70, 90, 75, 95] fig = px.scatter(x=study_hours, y=exam_scores, text=students, labels={'x': 'Study Hours', 'y': 'Exam Scores'}) fig.update_traces(marker=dict(size=12, line=dict(width=2, color='DarkSlateGrey')), selector=dict(mode='markers+text')) fig.update_layout(title='Study Hours vs. Exam Scores', xaxis_title='Study Hours', yaxis_title='Exam Scores') fig.show()
In this example, we use Plotly Express (px) to create a scatter plot. We define the x-axis as study hours, the y-axis as exam scores, and use the text parameter to display the students’ names as labels on the data points. We also customize the marker size and line properties for better visibility.
Interactive Bar Charts
Plotly allows us to create interactive bar charts as well. Let’s visualize the monthly sales of a company using an interactive bar chart:
python import plotly.graph_objects as go months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] sales = [15000, 18000, 12000, 17000, 20000, 22000, 19000, 21000, 24000, 23000, 25000, 28000] fig = go.Figure([go.Bar(x=months, y=sales)]) fig.update_layout(title='Monthly Sales', xaxis_title='Months', yaxis_title='Sales', showlegend=False) fig.show()
Here, we utilize the go.Bar() function from Plotly’s Graph Objects (go) to create an interactive bar chart. The fig.update_layout() function customizes the chart title and axes labels, while showlegend=False hides the legend.
Interactive Pie Charts
We can create interactive pie charts using Plotly as well. Let’s visualize the market share distribution for a company interactively:
python import plotly.graph_objects as go markets = ['Market A', 'Market B', 'Market C', 'Market D'] market_shares = [30, 25, 15, 30] fig = go.Figure(data=[go.Pie(labels=markets, values=market_shares)]) fig.update_traces(hoverinfo='label+percent', textinfo='value', textfont_size=15) fig.update_layout(title='Market Share Distribution') fig.show()
In this code snippet, we create an interactive pie chart using go.Pie(). We set hoverinfo to display both the label and percentage when hovering over a section of the pie. The textinfo parameter displays the exact value of each market share. Customization of the chart’s title and appearance is done using fig.update_layout().
Adding Additional Interactivity
Interactive charts become even more engaging when additional interactivity is added. Let’s explore some common interactive features that can be incorporated into your charts.
Hover Effects
Adding hover effects provides extra information when the user hovers over data points. Let’s modify the scatter plot we created earlier to include hover text:
python import plotly.express as px students = ['Alice', 'Bob', 'Charlie', 'David', 'Eve'] study_hours = [5, 3, 6, 4, 7] exam_scores = [85, 70, 90, 75, 95] fig = px.scatter(x=study_hours, y=exam_scores, text=students, labels={'x': 'Study Hours', 'y': 'Exam Scores'}, hover_name=students, hover_data={'study_hours': True, 'exam_scores': True}) fig.update_traces(marker=dict(size=12, line=dict(width=2, color='DarkSlateGrey')), selector=dict(mode='markers+text')) fig.update_layout(title='Study Hours vs. Exam Scores with Hover Effects', xaxis_title='Study Hours', yaxis_title='Exam Scores', hovermode='closest') fig.show()
In this modified scatter plot, we use the hover_name and hover_data parameters to display additional information on hover. When the user hovers over a data point, it will show the student’s name, study hours, and exam scores.
Zooming and Panning
Implementing zooming and panning capabilities in charts allows users to focus on specific data ranges and explore details. Let’s enable zooming and panning for the interactive bar chart:
python import plotly.graph_objects as go months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] sales = [15000, 18000, 12000, 17000, 20000, 22000, 19000, 21000, 24000, 23000, 25000, 28000] fig = go.Figure([go.Bar(x=months, y=sales)]) fig.update_layout(title='Monthly Sales with Zooming and Panning', xaxis_title='Months', yaxis_title='Sales', xaxis=dict(type='category'), yaxis=dict(autorange=True), dragmode='zoom', hovermode='x') fig.show()
In this code, we set the xaxis to type ‘category’ to ensure the x-axis displays the categorical data correctly. Additionally, yaxis=dict(autorange=True) allows the y-axis to auto-scale based on the data.
We enable zooming by setting dragmode=’zoom’. When users drag the mouse over the chart, it will zoom in on the selected area. The hovermode=’x’ ensures that hover effects are only activated along the x-axis.
Deploying Interactive Charts
Once you’ve created your interactive charts using Matplotlib or Plotly, you may want to share them with others. Several options allow you to do this:
- Saving Locally: You can save the chart as an image or HTML file on your local machine. For example:
python # Saving an interactive Plotly chart as an HTML file fig.write_html('interactive_chart.html')
- Embedding: Interactive Plotly charts can be embedded in web applications, blog posts, or documentation. Use the plotly.io.to_html() function to get the HTML code for embedding:
python import plotly.io as pio # Get the HTML code for embedding the chart chart_html = pio.to_html(fig)
- Online Hosting: You can upload your interactive Plotly charts to Plotly’s Chart Studio (https://chart-studio.plotly.com/) for online hosting and easy sharing.
- Jupyter Notebooks: If you’re working with Jupyter Notebooks, you can directly display the charts in the notebook using fig.show() for Plotly or plt.show() for Matplotlib.
Conclusion
Data visualization is a powerful technique for presenting complex information in an easily digestible manner. Python, with libraries like Matplotlib and Plotly, offers developers versatile options for creating both static and interactive charts.
In this blog post, we covered the basics of using Python functions to build static charts with Matplotlib and enhancing them with interactivity using Plotly. We explored different chart types, such as line charts, bar charts, and pie charts, and learned how to add hover effects, zooming, and panning to enhance the user experience.
With these skills, you can create compelling visualizations that not only communicate data insights effectively but also engage your audience with interactive elements. As you continue to explore data visualization in Python, you’ll unlock countless possibilities for conveying information and gaining valuable insights from your data. Happy charting!
Table of Contents
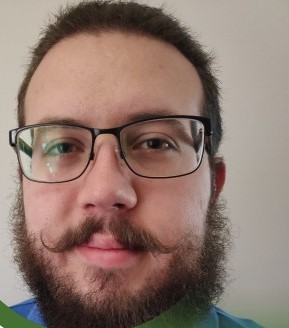
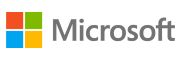