Python Generators: Navigating Large Data with Ease and Efficiency
Python, as a high-level, general-purpose programming language, boasts a wide variety of features that make it incredibly versatile and powerful, which is why many companies choose to hire Python developers. One such feature that Python developers leverage is the concept of ‘generators.’ In this blog post, we will take a deep dive into what generators are, how they work, and explore practical examples of their usage.
What are Generators?
Generators are a unique type of iterator, a broad category of objects in Python that allow us to iterate over the elements they contain. While lists, tuples, and other data types are also iterators, generators stand apart due to their ability to generate values on the fly, using a process called ‘lazy evaluation.’ This means they don’t store all the values in memory; they generate them as they go.
In contrast, a list or a tuple will store all its values in memory at once. This can become a problem when dealing with large volumes of data. Enter generators, which use a function rather than a data structure to iteratively produce values, only generating the next value when required.
How are Generators Defined?
Generators in Python are defined similarly to a function, but instead of the `return` statement, they use the `yield` keyword. The `yield` statement pauses the function, saving its state, and later resumes from where it left off when next called. This is what enables a generator to produce a sequence of results over time, rather than computing them at once and sending them back like a list.
Here’s a simple example of a generator:
```python def simple_generator(): yield 1 yield 2 yield 3 # Create a generator object gen = simple_generator() # Iterate over the generator object for number in gen: print(number) ```
In this case, the output will be:
``` 1 2 3 ```
Practical Examples of Generators
Let’s examine some examples to better illustrate the uses and benefits of generators.
Example 1: Generating Fibonacci Series
The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. Here’s how we can use a generator to produce the Fibonacci series:
```python def fibonacci(limit): a, b = 0, 1 while a < limit: yield a a, b = b, a + b # Create a generator object for Fibonacci series up to 100 fib = fibonacci(100) # Print the Fibonacci series for num in fib: print(num) ```
Example 2: Reading Large Files
When reading large files, loading the entire file into memory can be a problem. With generators, we can read the file line by line, reducing memory usage.
```python def read_large_file(file_path): with open(file_path, 'r') as file: for line in file: yield line # Create a generator object for reading a large file file_gen = read_large_file('large_file.txt') # Print each line in the large file for line in file_gen: print(line) ```
Example 3: Generating an Infinite Sequence
Generators can produce infinite sequences. Here’s an example:
```python def infinite_sequence(): num = 0 while True: yield num num += 1 # Create a generator object for the infinite sequence inf_seq = infinite_sequence() # Print the first 10 numbers of the infinite sequence for i in range(10): print(next(inf_seq)) ```
Performance Advantage of Generators
Generators are not only useful for their ability to handle large datasets without loading them into memory, they can also offer significant performance improvements. Let’s illustrate this with an example.
We’ll create a list and a generator, both producing the numbers from 0 to 1,000,000. We will then measure the time it takes to sum these numbers using the built-in `sum` function.
```python import time # List of 1,000,000 numbers start_time = time.time() num_list = [i for i in range(1, 1000001)] print(f"Sum of list: {sum(num_list)}") print(f"Time taken for list: {time.time() - start_time}") # Generator for 1,000,000 numbers start_time = time.time() num_gen = (i for i in range(1, 1000001)) print(f"Sum of generator: {sum(num_gen)}") print(f"Time taken for generator: {time.time() - start_time}") ```
While the actual times may vary, you’ll generally find that the generator is faster than the list. This is because it doesn’t have to generate all the numbers at once and store them in memory; it just needs to keep track of where it is in the sequence and generate the next number when needed.
Conclusion
Generators in Python are a powerful tool that Python developers often use for dealing with large amounts of data or computations that could benefit from a ‘lazy evaluation’ approach. They are versatile and can offer performance improvements over more traditional data structures. That’s why businesses looking to optimize their data handling processes frequently hire Python developers. By understanding and using Python’s generator functions, these developers can write more efficient, scalable, and memory-friendly code.
Table of Contents
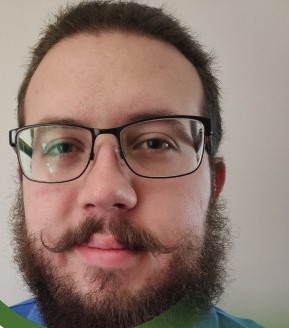
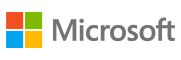