Python Tutorial: Understanding Exception Handling
Exception handling is a crucial aspect of writing robust and reliable Python programs. As a developer, you’ll inevitably encounter situations where your code might encounter unexpected errors or situations that can disrupt its normal execution. These disruptions, known as exceptions, can range from input validation errors to network connectivity issues. Understanding how to handle these exceptions gracefully is essential for producing stable and user-friendly applications.
Table of Contents
1. What are Exceptions?
In Python, an exception is an event that interrupts the normal flow of the program’s execution. These exceptions occur when the interpreter encounters an error that prevents it from continuing with its default behavior. These errors can arise due to various reasons, such as incorrect user input, file not found, or dividing by zero. Instead of crashing the program, Python provides a mechanism to catch and handle these exceptions, allowing you to provide an appropriate response or recovery process.
2. Common Types of Exceptions
Python comes with a variety of built-in exception types, each catering to a specific type of error. Some common exceptions include:
- SyntaxError: Raised when the Python interpreter encounters incorrect syntax in the code.
- TypeError: Occurs when an operation or function is applied to an object of inappropriate type.
- ValueError: Raised when a function receives an argument of correct type but with an inappropriate value.
- FileNotFoundError: Occurs when a file operation (like opening a file) is performed on a non-existent file.
- ZeroDivisionError: Raised when attempting to divide by zero.
- IndexError: Occurs when trying to access an index that is out of range in a sequence.
- KeyError: Raised when attempting to access a dictionary key that doesn’t exist.
3. The try-except Block: Handling Exceptions
Python provides a powerful construct called the try-except block to handle exceptions gracefully. The try block contains the code that might raise an exception, and the except block defines the code to execute when an exception occurs. This allows you to catch exceptions and take appropriate actions, such as displaying an error message or implementing a fallback strategy.
Here’s the basic structure of a try-except block:
python try: # Code that might raise an exception result = value / divisor except SomeException: # Code to handle the exception print("An error occurred")
In this example, if an exception of type SomeException is raised in the try block, the code within the corresponding except block will be executed.
3.1. Handling Specific Exceptions
You can handle specific exceptions by mentioning their types in the except clause. This allows you to provide targeted solutions for different types of errors.
python try: file = open("data.txt", "r") content = file.read() file.close() except FileNotFoundError: print("The file does not exist.") except PermissionError: print("You do not have permission to access this file.")
In this code snippet, the program attempts to open a file, read its content, and then close it. If the file is not found, a FileNotFoundError is raised, and the corresponding except block is executed. Similarly, if a PermissionError occurs, the appropriate message is displayed.
3.2. Handling Multiple Exceptions
You can also handle multiple exceptions using a single except block by specifying the exception types within a tuple.
python try: result = value / divisor except (ZeroDivisionError, ValueError): print("An error occurred")
In this example, both ZeroDivisionError and ValueError are caught by the same except block.
4. The else and finally Blocks
In addition to try and except, Python provides two more blocks that can be used with exception handling: else and finally.
4.1. The else Block
The else block is executed if no exceptions are raised in the try block. It’s useful for placing code that should run only if the try block executes successfully.
python try: result = value / divisor except ZeroDivisionError: print("Cannot divide by zero") else: print("Result:", result)
In this example, if no ZeroDivisionError occurs, the else block will be executed, printing the result.
4.2. The finally Block
The finally block is always executed, regardless of whether an exception occurred or not. It’s commonly used for releasing resources like closing files or network connections, ensuring that cleanup operations are performed.
python try: file = open("data.txt", "r") content = file.read() except FileNotFoundError: print("The file does not exist.") finally: file.close()
Here, the file is opened, and its content is read in the try block. If a FileNotFoundError occurs, the appropriate message is displayed. However, regardless of whether an exception occurred, the finally block ensures that the file is closed.
5. Raising Exceptions
As a developer, you can also raise exceptions intentionally to indicate errors or exceptional situations in your code. Python provides the raise statement for this purpose.
python def validate_age(age): if age < 0: raise ValueError("Age cannot be negative") if age > 120: raise ValueError("Invalid age") return age
In this example, the validate_age function raises a ValueError if the provided age is negative or greater than 120.
6. Custom Exceptions
Python allows you to define your own custom exception classes by inheriting from the Exception class. This is helpful when you want to create custom error types that are specific to your application.
python class CustomError(Exception): def __init__(self, message): self.message = message try: raise CustomError("This is a custom exception") except CustomError as ce: print("Caught CustomError:", ce.message)
Here, a CustomError class is defined, which takes a message as an argument. When this custom exception is raised and caught, the associated message is printed.
Conclusion
Exception handling is a fundamental skill for any Python developer. By effectively managing exceptions, you can create more reliable and user-friendly applications. Python’s try-except blocks, along with else and finally, provide powerful tools for gracefully handling errors and ensuring proper resource management. Whether you’re dealing with built-in exceptions or creating your own custom error types, mastering exception handling will undoubtedly elevate the quality of your Python programs. Remember, robust code isn’t just about functionality; it’s also about gracefully handling the unexpected.
In this tutorial, we’ve only scratched the surface of exception handling in Python. As you continue your coding journey, you’ll encounter a wide range of scenarios where understanding and implementing exception handling will be indispensable. Happy coding!
Table of Contents
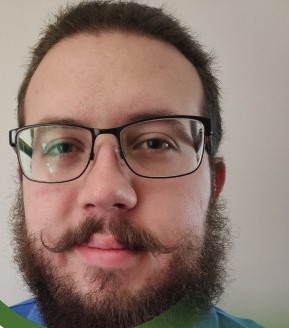
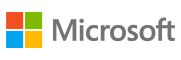