How to handle missing data in Python?
Handling missing data is a fundamental step in data preprocessing, especially when working with real-world datasets. In Python, the library most often used for this purpose is `pandas`, a powerful tool for data analysis and manipulation.
- Identifying Missing Data:
In `pandas`, missing data is primarily represented using the `NaN` (Not a Number) value. To detect these values in a DataFrame or Series, you can use the `isna()` or `isnull()` methods:
```python import pandas as pd df = pd.DataFrame({'A': [1, 2, np.nan], 'B': [4, np.nan, 6]}) missing_values = df.isna() ```
- Removing Missing Data:
If you want to remove rows or columns containing missing values, you can use the `dropna()` method:
```python df.dropna() # Removes rows with any missing values df.dropna(axis=1) # Removes columns with any missing values ```
- Imputing Missing Data:
Instead of removing missing data, you can also fill or “impute” them. The `fillna()` method is handy for this:
```python df.fillna(0) # Fill missing values with 0 df['A'].fillna(df['A'].mean()) # Fill missing values in column 'A' with the mean of 'A' ```
More advanced techniques can use methods like interpolation or even machine learning models to predict and fill in missing values.
- Interpolation:
This method can be used to fill missing values based on other values in the dataset. For instance, for a time-series data:
```python df.interpolate(method='linear') # Fills missing values using linear interpolation ```
Handling missing data is crucial to ensure the integrity and reliability of your analyses or models. The `pandas` library in Python offers a suite of tools for detecting, removing, and imputing missing values, allowing you to prepare your datasets effectively for subsequent processing or modeling. However, always choose the appropriate method based on the nature and distribution of your data, as well as the goal of your analysis.
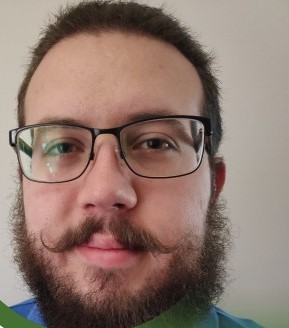
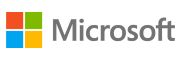