From Pixels to Pictures: A Deep Dive into Python Image Processing
As our world becomes increasingly digital, we have seen an explosion in the amount of visual data that we generate. From personal photographs to professional graphics, visual imagery is an integral part of how we communicate. However, managing and manipulating this data can be a challenge. This is where the skills of Python developers come into play, given their proficiency in leveraging Python’s numerous powerful tools for image processing. Whether you’re looking to understand these processes for your own knowledge or you’re looking to hire Python developers to optimize your visual data processing, this blog post will explore how Python functions can be effectively used for image processing tasks.
Python and Image Processing
Python, a high-level, object-oriented programming language, is particularly well-suited to image processing tasks due to its simplicity and the wide range of external libraries available. Some of the most popular libraries include PIL (Python Imaging Library), OpenCV (Open Source Computer Vision Library), and scikit-image.
Setting Up the Environment
Before we dive into examples, you need to have Python and the necessary libraries installed on your computer. You can download Python from the official website. After you have installed Python, you can use pip, Python’s package installer, to install the necessary libraries. Open your command line interface and type the following commands:
```python pip install numpy pip install pillow pip install opencv-python pip install scikit-image ```
Basic Image Manipulations
Let’s start with basic image manipulations like reading an image, resizing it, and saving it.
```python from PIL import Image # Open an image file img = Image.open('image.jpg') # Resize the image img = img.resize((800, 800)) # Save the image img.save('resized_image.jpg') ```
In the code above, we first import the Image module from the PIL library. We then open an image file, resize it to 800×800 pixels, and save the result.
Color Manipulations
Images are typically composed of pixels in three color channels: red, green, and blue (RGB). Python allows us to manipulate these color channels to achieve interesting effects.
Here’s an example of converting an image to grayscale:
```python # Convert the image to grayscale gray_img = img.convert('L') # Save the grayscale image gray_img.save('grayscale_image.jpg') ```
This code converts the image to grayscale (L stands for “luminance”) and saves the result.
Image Filtering
Python also allows us to apply filters to images, such as blurring or sharpening. Let’s take a look at an example of blurring an image:
```python from PIL import ImageFilter # Apply a blur filter blurred_img = img.filter(ImageFilter.BLUR) # Save the blurred image blurred_img.save('blurred_image.jpg') ```
In this example, we import the ImageFilter module from the PIL library, apply a blur filter to our image, and save the result.
Edge Detection
Edge detection is a common image processing task. It involves identifying points in an image where the brightness changes sharply, which usually corresponds to the edges of objects within the image.
Here’s how you can perform edge detection using the Canny edge detection method provided by the OpenCV library:
```python import cv2 import numpy as np # Read the image using OpenCV img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE) # Apply the Canny edge detection method edges = cv2.Canny(img, 100, 200) # Save the result cv2.imwrite('edges.jpg', edges) ```
In this example, we first read the image using the cv2.imread function. We then apply the Canny edge detection method using the cv2.Canny function and save the result.
Image Segmentation
Image segmentation is the process of dividing an image into multiple parts, often to isolate objects or regions of interest. Here’s how you can use the watershed segmentation method provided by the scikit-image library:
```python from skimage.feature import peak_local_max from skimage.segmentation import watershed from scipy import ndimage # Convert the image to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Apply the Sobel filter to get the image gradients gradient = ndimage.sobel(gray) # Find the local maxima in the gradient image coordinates = peak_local_max(gradient, min_distance=20, labels=gray) # Perform the watershed segmentation markers = ndimage.label(coordinates)[0] labels = watershed(-gradient, markers, mask=gray) # Save the result cv2.imwrite('segmented.jpg', labels) ```
This code first converts the image to grayscale. It then applies the Sobel filter to get the image gradients, finds the local maxima in the gradient image, and performs the watershed segmentation. The result is saved as ‘segmented.jpg’.
Conclusion
Image processing is a powerful tool with a wide range of applications, from simple tasks like resizing and color conversions to more complex tasks like edge detection and segmentation. As we have seen, Python’s simplicity and the breadth of available libraries make it an excellent choice for these tasks. This versatility and ease of use make Python developers highly sought after in many industries. Whether you’re looking to delve into the world of image processing with Python yourself or planning to hire Python developers for your project, the examples we have covered in this blog post should provide a good starting point.
Remember, these are just the basics. Python’s image processing capabilities extend far beyond what we have covered here. If you hire Python developers, they can help you experiment and explore these more advanced techniques, unlocking the full potential of what Python has to offer in the field of image processing!
Table of Contents
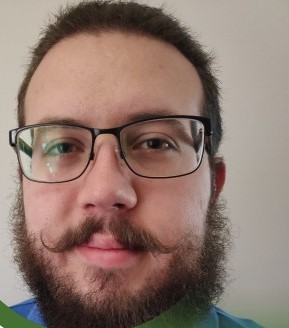
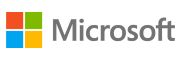