Python Q & A
How to implement encryption in Python?
Implementing encryption is essential for ensuring data confidentiality in various applications. Python provides a range of libraries to facilitate encryption and decryption of data.
- Symmetric Encryption with `cryptography`: The `cryptography` library is a popular choice for cryptographic operations in Python. For symmetric encryption, it offers the Fernet method, which is simple yet secure.
```python from cryptography.fernet import Fernet key = Fernet.generate_key() # Generate a key cipher_suite = Fernet(key) encrypted_text = cipher_suite.encrypt(b"Hello, World!") # Encrypt decrypted_text = cipher_suite.decrypt(encrypted_text) # Decrypt ```
- Asymmetric Encryption: `cryptography` also supports asymmetric encryption using public and private key pairs. The RSA method is commonly used.
```python from cryptography.hazmat.primitives.asymmetric import rsa from cryptography.hazmat.primitives import serialization # Generate private key and corresponding public key private_key = rsa.generate_private_key(public_exponent=65537, key_size=2048) public_key = private_key.public_key() # Serialize keys for storage pem_private = private_key.private_bytes(encoding=serialization.Encoding.PEM, format=serialization.PrivateFormat.PKCS8, encryption_algorithm=serialization.NoEncryption()) pem_public = public_key.public_bytes(encoding=serialization.Encoding.PEM, format=serialization.PublicFormat.SubjectPublicKeyInfo) ```
- Hashing: While not encryption, hashing is a related concept, used to securely store and verify data, such as passwords. Libraries like `bcrypt` are recommended for hashing passwords due to their resistance against brute-force attacks and their use of salting.
```python import bcrypt password = b"supersecretpassword" hashed = bcrypt.hashpw(password, bcrypt.gensalt()) # Hash a password bcrypt.checkpw(password, hashed) # Check a password against the hashed version ```
- Best Practices:
– Key Management: Safeguard encryption keys. If they’re compromised, encrypted data can be decrypted. Consider using key management solutions or services.
– Regularly Update: Cryptographic best practices evolve. What’s deemed secure today might be vulnerable tomorrow. Regularly update your libraries and practices.
Python offers powerful libraries like `cryptography` for encryption. Ensure you’re always following best practices to maintain the security of your encrypted data.
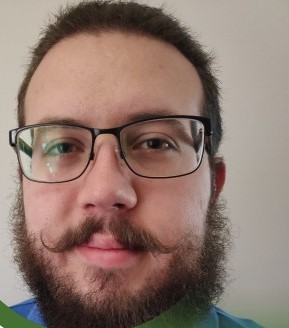
Previously at
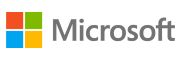
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git