Hire Python Developers Interview Questions Guide
Python, renowned for its versatility and readability, continues to be a preferred language for a multitude of applications. When seeking to develop robust software solutions, hiring skilled Python developers is paramount. This guide serves as your compass through the hiring voyage, empowering you to evaluate candidates’ technical prowess, problem-solving abilities, and Python expertise. Let’s set forth on this exploration, unveiling vital interview questions and strategies to pinpoint the perfect Python developer for your team.
Table of Contents
1. How to Hire Python Developers
Embarking on the quest to hire Python developers? Follow these steps for a successful journey:
- Job Requirements: Define specific job prerequisites, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ Python proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of Python Developers to Look For
When evaluating Python developers, be on the lookout for these core skills:
- Python Proficiency: A deep understanding of Python syntax, libraries, and coding conventions.
- Problem-Solving Abilities: An aptitude for identifying challenges and devising efficient solutions.
- Software Development: Proficiency in software development methodologies, version control, and collaborative tools.
- Web Development Skills: Familiarity with web frameworks like Django or Flask for building robust web applications.
- Database Expertise: Knowledge of working with databases, SQL, and data modeling.
- Data Science Skills: Expertise in data analysis, manipulation, and visualization using libraries like Pandas and Matplotlib.
- API Integration: Experience with integrating APIs and web services to enhance application functionality.
- Testing and Debugging: Ability to write unit tests and debug code to ensure software reliability.
3. Overview of the Python Developer Hiring Process
Here’s an overview of the Python developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create captivating job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting Python Developer Interview Questions
Develop a comprehensive set of interview questions covering Python intricacies, problem-solving aptitude, and relevant technologies.
4. Sample Python Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ Python skills:
Q1. Explain the Global Interpreter Lock (GIL) in Python. How does it affect multi-threading?
A: The GIL is a mutex that prevents multiple native threads from executing Python code concurrently in the same process. It impacts multi-threading as only one thread can execute Python bytecode at a time, limiting the performance gain from multi-threading in CPU-bound tasks.
Q2. Implement a Python function that calculates the Fibonacci sequence using recursion.
def fibonacci(n): if n <= 0: return [] elif n == 1: return [0] elif n == 2: return [0, 1] else: fib_sequence = fibonacci(n - 1) fib_sequence.append(fib_sequence[-1] + fib_sequence[-2]) return fib_sequence
Q3. Explain the purpose of a virtual environment in Python. How does it help manage dependencies?
A: A virtual environment is an isolated Python environment that allows you to manage dependencies and packages separately for different projects. It prevents conflicts between packages and ensures consistent and reproducible environments.
Q4. Build a Python class that represents a basic bank account with methods for depositing and withdrawing funds.
class BankAccount: def __init__(self, balance=0): self.balance = balance def deposit(self, amount): self.balance += amount def withdraw(self, amount): if self.balance >= amount: self.balance -= amount else: print("Insufficient balance") def get_balance(self): return self.balance
Q5. What is the purpose of Python decorators? Provide an example of using a decorator to measure function execution time.
A: Decorators are functions that modify or enhance the behavior of other functions or methods. Here’s an example of a decorator to measure execution time:
import time def measure_time(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) end_time = time.time() print(f"{func.__name__} took {end_time - start_time} seconds") return result return wrapper @measure_time def example_function(): time.sleep(2) example_function()
Q6. Explain the purpose of Python’s “try…except” statement and how it handles exceptions.
A: The try...except
statement is used to handle exceptions and prevent program crashes. Code within the try
block is executed, and if an exception occurs, it’s caught by the corresponding except
block.
Q7. Implement a Python generator that yields the squares of numbers up to a given limit.
def squares_generator(limit): for i in range(limit): yield i ** 2
Q8. Explain the difference between a shallow copy and a deep copy in Python.
A: A shallow copy of an object creates a new object with a new reference, but the elements inside the object are still references to the same objects as the original. A deep copy creates a new object and recursively copies all objects referenced by the original.
Q9. Write a Python function that uses list comprehension to filter even numbers from a list.
def filter_even_numbers(numbers): return [num for num in numbers if num % 2 == 0]
Q10. What is a lambda function in Python? Provide an example of using a lambda function to sort a list of tuples based on the second element.
A: A lambda function is an anonymous function defined using the lambda
keyword. Here’s an example:
data = [(1, 5), (2, 3), (3, 8)] sorted_data = sorted(data, key=lambda x: x[1])
5. Hiring Python Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected Python developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional Python developers, ensuring your team possesses the skills required to build remarkable software solutions.
6. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess Python developers comprehensively. Whether you’re developing web applications or data analysis tools, securing the right Python developers for your team is pivotal to the success of your projects.
Table of Contents
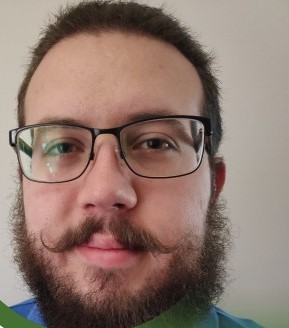
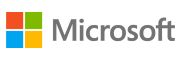