How to iterate over a dictionary in Python?
Iterating over dictionaries is a common operation in Python, and the language provides several concise and readable methods to facilitate this. A dictionary, as you might know, consists of key-value pairs. Depending on your specific needs, you might want to iterate over just the keys, just the values, or both.
- Iterating Over Keys:
By default, when you loop through a dictionary using a `for` loop, you’re iterating over its keys:
```python for key in my_dict: print(key) ```
Alternatively, for clarity, you can use the `keys()` method:
```python for key in my_dict.keys(): print(key) ```
- Iterating Over Values:
If you’re only interested in the values stored in the dictionary and not the keys, you can use the `values()` method:
```python for value in my_dict.values(): print(value) ```
- Iterating Over Key-Value Pairs:
Often, you’ll want to access both the key and its corresponding value while iterating. The `items()` method makes this convenient by returning pairs of keys and values:
```python for key, value in my_dict.items(): print(key, value) ```
A point to remember is that as of Python 3.7, dictionaries maintain the insertion order of their items. This means that when you iterate over a dictionary, you’ll get the key-value pairs in the order they were added. However, in versions of Python before 3.7, dictionaries didn’t guarantee any specific order.
Dictionaries in Python provide intuitive methods to iterate over their content, whether you want to access the keys, the values, or both. The choice of method (`keys()`, `values()`, or `items()`) depends on the specific requirements of your task. Familiarity with these methods is essential for anyone working with dictionaries in Python, ensuring code that’s both efficient and easily readable.
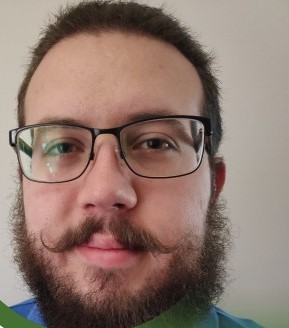
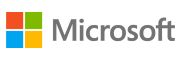