Python Q & A
How to handle large datasets in Python?
Handling large datasets in Python requires a combination of efficient tools and best practices to ensure performance and accuracy. Here’s how to approach it:
- Use Pandas with Dask: While Pandas is an excellent library for data manipulation, it loads the entire dataset into memory, which may not be feasible for very large datasets. Dask extends Pandas to allow operations on larger-than-memory datasets by breaking them into smaller chunks and processing these chunks in parallel.
- Database Integration: Instead of loading the whole dataset into memory, consider using databases like PostgreSQL or SQLite to store and query your data. Tools like SQLAlchemy or Pandas itself can help interface with these databases directly.
- Memory-Efficient Data Types: In Pandas, choose appropriate data types. For example, use category dtype for columns with a limited set of values instead of object dtype. Also, for numerical columns, if possible, use int8, int16, or float32 instead of the default int64 or float64 to save memory.
- Chunk Processing: If you’re reading from flat files like CSVs, use the `chunksize` parameter in Pandas’ `read_csv` method. This reads the file in chunks, allowing you to process datasets that are larger than available memory.
- Optimize Computations: Use vectorized operations provided by libraries like NumPy or Pandas instead of Python loops. These operations are internally optimized and can handle large data much faster.
- Sampling and Aggregations: Instead of working on the entire dataset, consider sampling a subset for exploratory data analysis. Also, use aggregation methods to reduce the size of the dataset by grouping similar data.
- Parallel Processing: Use libraries like `joblib` or `multiprocessing` to leverage multi-core CPUs. This can significantly speed up computations on large datasets.
- Utilize Cloud Solutions: Platforms like Google BigQuery or AWS Redshift allow for manipulation and querying of massive datasets without the need to download or store them locally.
Handling large datasets in Python is a combination of selecting the right tools and adopting best practices. By being mindful of memory constraints and optimizing for performance, you can efficiently process and derive insights from vast amounts of data.
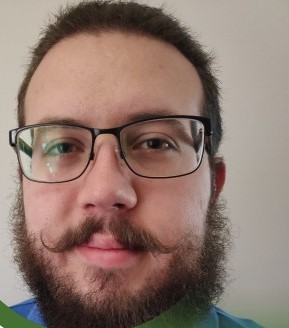
Previously at
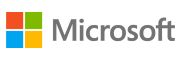
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git