10 Python Libraries for Audio Processing
Audio processing has become an essential component in various fields such as music production, speech recognition, audio analysis, and more. Python, with its user-friendly syntax and extensive libraries, has become a popular choice for audio processing tasks. Whether you’re a musician, a data scientist, or an enthusiast, these 10 Python libraries for audio processing will help you explore and manipulate sound in creative and efficient ways.
Table of Contents
Introduction: The Power of Python in Audio Processing
Python’s versatility extends to audio processing, offering an array of libraries that enable developers to perform various operations on audio data. From basic tasks like reading and writing audio files to more advanced tasks like applying complex filters and conducting spectral analysis, Python libraries have you covered. Let’s dive into 10 such libraries that stand out for their capabilities and ease of use.
1. NumPy and SciPy: Foundational Tools
NumPy and SciPy are fundamental libraries in the Python scientific computing ecosystem. While not exclusively designed for audio processing, they play a pivotal role in handling and manipulating audio data. NumPy provides support for multi-dimensional arrays, which are ideal for representing audio signals. SciPy, built on top of NumPy, offers signal processing functions, including filtering, convolution, and more.
Code Sample: Reading and Plotting an Audio File
python import numpy as np import matplotlib.pyplot as plt from scipy.io import wavfile # Load audio file sample_rate, audio_data = wavfile.read('sample_audio.wav') # Plot audio waveform plt.figure(figsize=(10, 4)) plt.plot(np.arange(len(audio_data)) / sample_rate, audio_data) plt.xlabel('Time (s)') plt.ylabel('Amplitude') plt.title('Audio Waveform') plt.show()
2. librosa: Music and Audio Analysis
Librosa is a specialized library for analyzing and extracting features from music and audio signals. It’s widely used in music information retrieval, genre classification, beat detection, and more. Librosa offers functions to compute spectrograms, chromagrams, and mel-frequency cepstral coefficients (MFCCs), making it an excellent choice for music-related projects.
Code Sample: Extracting Mel-Frequency Cepstral Coefficients (MFCCs)
python import librosa import librosa.display import matplotlib.pyplot as plt # Load audio file audio_file = 'sample_music.wav' y, sr = librosa.load(audio_file) # Extract MFCCs mfccs = librosa.feature.mfcc(y=y, sr=sr) # Display MFCCs plt.figure(figsize=(10, 4)) librosa.display.specshow(mfccs, x_axis='time') plt.colorbar(format='%+2.0f dB') plt.title('MFCCs') plt.show()
3. soundfile: Reading and Writing Sound Files
The soundfile library simplifies the process of reading and writing various audio file formats. It provides a consistent interface for different formats and supports high-quality audio I/O. This library is a valuable addition when dealing with audio data from diverse sources.
Code Sample: Reading and Writing Audio Files with soundfile
python import soundfile as sf # Read audio file data, sample_rate = sf.read('input_audio.wav') # Write audio file sf.write('output_audio.wav', data, sample_rate)
4. pydub: Simplifying Audio Manipulation
Pydub is a user-friendly library that simplifies audio manipulation tasks. It provides a high-level interface for various audio operations, such as slicing, concatenation, and applying effects. Pydub’s ease of use makes it a great choice for beginners in audio processing.
Code Sample: Concatenating Audio Files with pydub
python from pydub import AudioSegment # Load audio files audio1 = AudioSegment.from_file('audio1.wav') audio2 = AudioSegment.from_file('audio2.wav') # Concatenate audio files combined_audio = audio1 + audio2 # Export concatenated audio combined_audio.export('combined_audio.wav', format='wav')
5. pyAudio: Real-time Audio Processing
pyAudio is a library that enables real-time audio input and output. It’s particularly useful for creating applications that require audio streaming, such as voice chat, audio synthesis, and real-time audio effects. pyAudio provides a simple interface to interact with audio devices.
Code Sample: Recording and Playing Audio in Real-Time with pyAudio
python import pyaudio import numpy as np # Initialize pyAudio p = pyaudio.PyAudio() # Open audio stream stream = p.open(format=pyaudio.paFloat32, channels=1, rate=44100, input=True, output=True) # Record and play audio in real-time frames_per_buffer = 1024 for _ in range(100): audio_data = np.random.randn(frames_per_buffer).astype(np.float32) stream.write(audio_data.tobytes()) # Close the stream and terminate pyAudio stream.stop_stream() stream.close() p.terminate()
6. simpleaudio: Cross-platform Audio Playback
Simpleaudio provides a straightforward way to play audio files across different platforms. It supports various audio formats and offers a hassle-free solution for adding audio playback to your Python applications.
Code Sample: Playing an Audio File with simpleaudio
python import simpleaudio as sa # Load audio file wave_obj = sa.WaveObject.from_wave_file('sample_audio.wav') # Play audio play_obj = wave_obj.play() play_obj.wait_done()
7. audioread: Cross-format Audio Decoding
The audioread library is a simple tool for decoding audio files of various formats. It’s useful when you need to read audio data from different file types without worrying about format-specific details.
Code Sample: Reading Audio Files with audioread
python import audioread # Open audio file with audioread.audio_open('audio_file.mp3') as f: print(f.channels, f.samplerate) for buf in f: process_audio_buffer(buf)
8. madmom: Music Information Retrieval
Madmom is a library focused on music information retrieval (MIR). It offers tools for beat and tempo estimation, onset detection, and other MIR tasks. Madmom simplifies complex music analysis processes and is widely used in music research.
Code Sample: Beat Tracking with madmom
python from madmom.features.beats import BeatTrackingProcessor, RNNBeatProcessor from madmom.audio.signal import Signal # Load audio signal audio_signal = Signal('music_track.wav') # Create beat tracking processor beat_processor = BeatTrackingProcessor(fps=100) # Track beats beats = beat_processor(audio_signal) print(beats)
9. pyo: Audio Synthesis and Processing
Pyo is a library designed for audio synthesis and signal processing. It provides a platform for creating interactive audio applications, sound design, and live performances. Pyo’s unique approach is centered around building audio networks and processing chains.
Code Sample: Creating an Audio Synthesis Patch with pyo
python from pyo import * # Initialize audio server s = Server().boot() # Create oscillator oscillator = Sine(freq=440, mul=0.1) # Start audio processing oscillator.out() # Sleep for 5 seconds s.sleep(5) # Stop audio processing s.stop()
10. TensorFlow and PyTorch: Deep Learning for Audio
While not exclusive audio libraries, TensorFlow and PyTorch, popular deep learning frameworks, can be utilized for advanced audio processing tasks. They enable tasks like speech recognition, sound generation, and audio classification using neural networks.
Code Sample: Sound Generation with a Neural Network in TensorFlow
python import tensorflow as tf # Define a simple generator model generator = tf.keras.Sequential([ tf.keras.layers.Dense(256, activation='relu', input_shape=(100,)), tf.keras.layers.Dense(512, activation='relu'), tf.keras.layers.Dense(1024, activation='relu'), tf.keras.layers.Dense(16384, activation='tanh'), tf.keras.layers.Reshape((128, 128, 1)) ]) # Generate a sample sound random_input = tf.random.normal((1, 100)) generated_sound = generator(random_input) # Play the generated sound
Conclusion
These 10 Python libraries open the doors to a vast world of audio processing possibilities. Whether you’re a musician seeking to manipulate sounds creatively or a data scientist working on advanced audio analysis, these libraries provide the tools you need. From foundational libraries like NumPy and SciPy to specialized ones like librosa and pyAudio, you have the power to explore, experiment, and innovate with audio in Python. So, dive in and start exploring the symphony of sound waiting to be discovered through these remarkable libraries.
Table of Contents
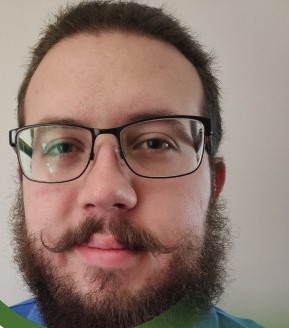
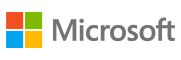