10 Python Libraries for Computer Vision
In today’s digital age, computer vision has become an integral part of various industries, from healthcare to automotive to entertainment. Python, with its rich ecosystem of libraries, has emerged as a popular choice for implementing computer vision tasks. These libraries provide developers with the tools and resources needed to process, analyze, and manipulate visual data efficiently. In this article, we will delve into 10 essential Python libraries for computer vision, each serving a unique purpose and catering to different aspects of the field.
Table of Contents
1. OpenCV (Open Source Computer Vision Library)
OpenCV is the go-to library for computer vision tasks. It boasts a vast collection of algorithms and functions that facilitate tasks such as image and video processing, feature extraction, object detection, and more. Its simple interface, extensive documentation, and compatibility with various platforms make it a preferred choice for both beginners and experts in the field.
Code Sample:
python import cv2 # Read an image from file image = cv2.imread('image.jpg') # Convert the image to grayscale gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Display the original and grayscale images cv2.imshow('Original Image', image) cv2.imshow('Grayscale Image', gray_image) cv2.waitKey(0) cv2.destroyAllWindows()
2. Dlib
Dlib is a versatile library that excels in face detection, facial landmark detection, image alignment, and more. It offers pre-trained models and tools for various machine learning tasks, making it a valuable asset for computer vision projects requiring accurate facial analysis.
Code Sample:
python import dlib import cv2 # Load a pre-trained face detection model detector = dlib.get_frontal_face_detector() # Load an image image = cv2.imread('face.jpg') # Detect faces in the image faces = detector(image) # Draw bounding boxes around detected faces for face in faces: x, y, w, h = face.left(), face.top(), face.width(), face.height() cv2.rectangle(image, (x, y), (x + w, y + h), (255, 0, 0), 2) cv2.imshow('Detected Faces', image) cv2.waitKey(0) cv2.destroyAllWindows()
3. Pillow
Pillow (PIL Fork) is a powerful library for image processing tasks. It supports various image formats and provides functionalities such as resizing, cropping, filtering, and adding text to images. Whether you’re working with photographs or generating visual content, Pillow offers an array of tools to manipulate images effectively.
Code Sample:
python from PIL import Image, ImageFilter # Open an image file image = Image.open('image.jpg') # Apply a blur filter blurred_image = image.filter(ImageFilter.BLUR) # Resize the image resized_image = image.resize((800, 600)) # Display the original, blurred, and resized images image.show() blurred_image.show() resized_image.show()
4. scikit-image
scikit-image is a user-friendly library for image processing and computer vision tasks. It provides a wide range of algorithms for tasks such as image segmentation, feature extraction, and morphological operations. With scikit-image, you can perform advanced manipulations on images without delving into complex mathematical details.
Code Sample:
python from skimage import io, color from skimage.feature import corner_harris, corner_peaks import matplotlib.pyplot as plt # Load an image image = io.imread('building.jpg') # Convert the image to grayscale gray_image = color.rgb2gray(image) # Detect corners using Harris corner detector corners = corner_peaks(corner_harris(gray_image), min_distance=5) # Display the original image with detected corners plt.imshow(image) plt.scatter(corners[:, 1], corners[:, 0], color='red') plt.show()
5. TensorFlow and Keras
TensorFlow and Keras are widely used libraries for machine learning, but they also offer excellent support for computer vision tasks. TensorFlow provides pre-trained models like Inception and ResNet for image classification, while Keras simplifies the process of building, training, and evaluating deep learning models.
Code Sample (using Keras for image classification):
python from tensorflow.keras.applications import InceptionV3 from tensorflow.keras.applications.inception_v3 import preprocess_input, decode_predictions from tensorflow.keras.preprocessing import image import numpy as np # Load the InceptionV3 model model = InceptionV3(weights='imagenet') # Load and preprocess an image img_path = 'image.jpg' img = image.load_img(img_path, target_size=(299, 299)) x = image.img_to_array(img) x = np.expand_dims(x, axis=0) x = preprocess_input(x) # Make predictions using the model predictions = model.predict(x) decoded_predictions = decode_predictions(predictions, top=5)[0] # Print the top predicted labels for label, description, score in decoded_predictions: print(f'{label}: {description} ({score:.2f})')
6. PyTorch and torchvision
Similar to TensorFlow and Keras, PyTorch and torchvision offer powerful tools for computer vision tasks. PyTorch’s dynamic computation graph and torchvision’s datasets and pre-trained models make it easy to implement tasks such as image classification, object detection, and style transfer.
Code Sample (using PyTorch and torchvision for image classification):
python import torch import torchvision.transforms as transforms import torchvision.models as models from torchvision import datasets # Load a pre-trained ResNet model model = models.resnet18(pretrained=True) model.eval() # Preprocess an image preprocess = transforms.Compose([ transforms.Resize(256), transforms.CenterCrop(224), transforms.ToTensor(), transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]), ]) # Load and preprocess an image img = Image.open('image.jpg') img_tensor = preprocess(img) img_tensor = torch.unsqueeze(img_tensor, 0) # Make predictions using the model with torch.no_grad(): predictions = model(img_tensor) # Print the top predicted label _, idx = torch.max(predictions, 1) print(f'Predicted label index: {idx.item()}')
7. SimpleCV
SimpleCV is designed to simplify computer vision tasks by providing an intuitive interface for image analysis and manipulation. It supports features like image filtering, feature detection, and interactive GUI-based tools for experimentation and visualization.
Code Sample:
python from SimpleCV import Image # Load an image img = Image('image.jpg') # Convert the image to grayscale gray_img = img.grayscale() # Apply edge detection edges = gray_img.edges() # Display the original, grayscale, and edge-detected images img.show() gray_img.show() edges.show()
8. imgaug
imgaug is a versatile library for augmenting images, a crucial step in training robust computer vision models. It provides an array of transformation techniques like rotation, scaling, flipping, and more. This library helps you increase the diversity of your training data and improve model generalization.
Code Sample:
python import imgaug.augmenters as iaa import imageio # Load an image image = imageio.imread('image.jpg') # Define augmentation pipeline augmenter = iaa.Sequential([ iaa.Fliplr(0.5), # Horizontal flips iaa.Crop(percent=(0, 0.1)), # Crop images by up to 10% iaa.GaussianBlur(sigma=(0, 3.0)) # Apply Gaussian blur with random sigma ]) # Augment the image augmented_image = augmenter.augment_image(image) # Display the original and augmented images plt.imshow(image) plt.show() plt.imshow(augmented_image) plt.show()
9. Caffe
Caffe is a deep learning framework known for its speed and efficiency in image classification tasks. It comes with a model zoo containing pre-trained models for various image-related tasks. While it’s slightly less user-friendly than some other libraries, its performance makes it a valuable asset for high-speed image processing applications.
10. Mahotas
Mahotas is a computer vision library that focuses on speed and efficient memory usage. It includes a variety of features for image processing, such as edge detection, texture analysis, and feature extraction. Mahotas is particularly useful for projects requiring real-time image analysis.
Conclusion
These 10 Python libraries cover a wide range of computer vision tasks, from image manipulation and analysis to deep learning and augmentation. Depending on the specific requirements of your project, you can choose the library that best suits your needs. Whether you’re a beginner exploring the world of computer vision or an experienced developer looking to streamline your workflow, these libraries will undoubtedly enhance your capabilities and empower you to create impressive visual applications.
Table of Contents
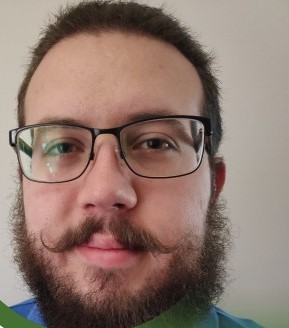
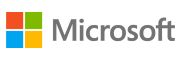