10 Python Libraries for Web Development
Python has emerged as one of the most popular programming languages for web development due to its simplicity, readability, and extensive community support. One of the reasons for its popularity is the wide range of powerful libraries available for web development tasks. These libraries enable developers to build robust and feature-rich web applications with ease. In this blog, we’ll explore 10 essential Python libraries that every web developer should know about. Whether you’re working on the frontend or the backend, these libraries will undoubtedly enhance your productivity and help you create stunning web experiences.
1. Flask – The Micro Web Framework:
Flask is a lightweight and flexible micro web framework that allows developers to build web applications with minimal boilerplate code. It provides essential features for routing, request handling, and templating, making it an excellent choice for small to medium-sized projects. Below is a simple example of a “Hello World” Flask application:
python from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run()
2. Django – The Batteries-Included Web Framework:
Django is a full-featured web framework that follows the “batteries-included” philosophy. It provides everything you need to build complex, database-driven web applications. Django’s ORM (Object-Relational Mapping) system simplifies database interactions, while its built-in admin interface allows for easy content management. Here’s a simple example of a Django model:
python from django.db import models class Book(models.Model): title = models.CharField(max_length=100) author = models.CharField(max_length=50) published_year = models.IntegerField() def __str__(self): return self.title
3. BeautifulSoup – Web Scraping Made Easy:
BeautifulSoup is a Python library that allows you to parse and extract data from HTML and XML documents. It’s a handy tool for web scraping tasks, such as extracting information from websites or parsing HTML pages. Here’s an example of using BeautifulSoup to extract all the links from a webpage:
python from bs4 import BeautifulSoup import requests url = 'https://example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') links = soup.find_all('a') for link in links: print(link.get('href'))
4. Requests – HTTP Library for Humans:
Requests is a user-friendly HTTP library that simplifies making HTTP requests in Python. Whether you’re sending GET requests or handling complex data with POST requests, Requests makes it easy. Below is a simple example of making a GET request using the Requests library:
python import requests url = 'https://api.example.com/data' response = requests.get(url) if response.status_code == 200: data = response.json() print(data)
5. SQLAlchemy – The SQL Toolkit and Object-Relational Mapper:
SQLAlchemy is a powerful and flexible SQL toolkit that provides a full suite of tools for working with databases in Python. It also includes an Object-Relational Mapper (ORM) that allows developers to interact with databases using Python classes. Here’s an example of defining a simple SQLAlchemy model:
python from sqlalchemy import Column, Integer, String, create_engine from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) email = Column(String) # Create an SQLite database in memory engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
6. Flask-RESTful – Building REST APIs Made Simple:
Flask-RESTful is an extension for Flask that makes it easy to build RESTful APIs. It provides abstractions for defining resources and handling HTTP methods, allowing you to create APIs quickly and efficiently. Here’s an example of defining a simple API using Flask-RESTful:
python from flask import Flask from flask_restful import Resource, Api app = Flask(__name__) api = Api(app) class HelloWorld(Resource): def get(self): return {'message': 'Hello, World!'} api.add_resource(HelloWorld, '/') if __name__ == '__main__': app.run()
7. Jinja2 – Templating Engine for Python:
Jinja2 is a popular templating engine for Python that allows you to generate dynamic content in web applications. It provides a simple and flexible syntax for defining templates and rendering data into HTML or other formats. Here’s a simple example of using Jinja2 to render a template with dynamic data:
python from jinja2 import Template template_str = 'Hello, {{ name }}!' template = Template(template_str) rendered = template.render(name='Alice') print(rendered) # Output: Hello, Alice!
8. Celery – Distributed Task Queue:
Celery is a powerful library for handling distributed tasks and asynchronous processing in Python web applications. It allows you to offload time-consuming tasks to background workers, enhancing the responsiveness and scalability of your web app. Below is an example of using Celery to perform an asynchronous task:
python from celery import Celery app = Celery('tasks', broker='pyamqp://guest@localhost//') @app.task def add(x, y): return x + y
9. WTForms – Form Validation and Rendering:
WTForms is a flexible library for handling form validation and rendering in web applications. It provides a simple way to define forms and validate user input, helping you avoid common security pitfalls. Below is a basic example of using WTForms to create a simple login form:
python from wtforms import Form, StringField, PasswordField, validators class LoginForm(Form): username = StringField('Username', [validators.Length(min=4, max=25)]) password = PasswordField('Password', [validators.Length(min=6, max=35)])
10. Gunicorn – Python WSGI HTTP Server:
Gunicorn is a production-ready WSGI HTTP server that can run Python web applications. It’s a reliable choice for deploying Flask or Django applications to handle high loads and ensure smooth performance. To run a Flask app using Gunicorn, you can use the following command:
bash gunicorn app:app
Conclusion:
Python’s versatility in web development is amplified by the wealth of powerful libraries available to developers. From building microservices to creating complex web applications, these ten Python libraries cover a broad spectrum of web development needs. Whether you’re a beginner or an experienced developer, incorporating these libraries into your toolkit will undoubtedly elevate your web development skills and help you build outstanding web experiences. So go ahead, explore these libraries, and take your Python web development to new heights!
Table of Contents
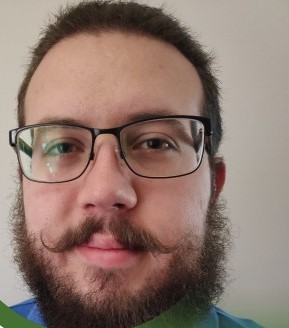
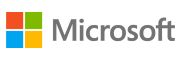