What is list comprehension in Python?
List comprehension is a concise and elegant way to create lists in Python. It provides a more syntactically readable approach to generate lists than using traditional loops. Essentially, list comprehension is a syntactic construct that allows for the creation of a new list by applying an expression to each item in an existing list (or other iterable objects), optionally filtering the results based on certain criteria.
Basic Syntax:
```python [expression for item in iterable if condition] ```
Examples:
- Creating a List of Squares:
```python squares = [x**2 for x in range(10)] print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] ```
- Filtering Results: Generate a list of even numbers from 0 to 9.
```python evens = [x for x in range(10) if x % 2 == 0] print(evens) # Output: [0, 2, 4, 6, 8] ```
- Nested List Comprehensions: Flatten a matrix into a single list.
```python matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flattened = [num for row in matrix for num in row] print(flattened) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9] ```
Benefits:
– Readability: List comprehensions can make code more readable, especially for simple transformations and filtering.
– Performance: They are often faster than equivalent `for` loops because of internal optimizations.
Caution:
While list comprehensions are powerful, they can reduce readability if overused or employed for complex operations. For more intricate scenarios, traditional loops might be a clearer choice.
List comprehensions are a hallmark of Pythonic code, offering a succinct way to generate lists. By understanding and using them effectively, developers can write cleaner, more efficient code while also making their programs more Pythonic in nature.
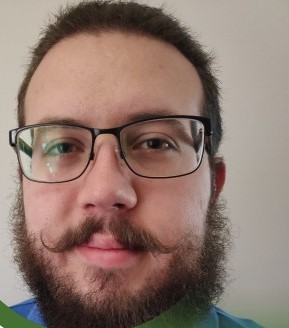
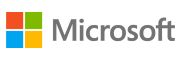