How to use list, set, and dictionary comprehensions in Python?
Comprehensions in Python offer a concise way to create lists, sets, and dictionaries. They provide syntactic sugar, allowing for clear and readable code. Let’s delve into each type:
- List Comprehensions:
A list comprehension provides a concise way to create lists. It consists of an expression followed by a `for` clause, and can have zero or more `if` clauses.
– Example: To create a list of the first ten square numbers:
```[x*x for x in range(10)]```
You can also filter results using conditions. For instance, to get squares of even numbers only:
```[x*x for x in range(10) if x % 2 == 0]```
- Set Comprehensions:
Similar to list comprehensions, but produces a set, which is an unordered collection with no duplicate entries.
– Example: To create a set of the first ten square numbers:
```{x*x for x in range(10)}```
Since sets inherently eliminate duplicates, this can be particularly useful when you want a collection of unique items.
- Dictionary Comprehensions:
Dictionary comprehensions allow you to express the creation of dictionaries using the same concise syntax. The key and value parts are separated by a colon in the expression.
– Example: To create a dictionary with numbers (up to nine) as keys and their squares as values:
```{x: x*x for x in range(10)}```
You can also conditionally produce key-value pairs similar to lists and sets.
- Beneficial Use Cases:
Comprehensions are especially powerful when working with existing collections, transforming them into new ones. They’re ideal for situations where creating a loop just to populate a collection feels verbose. By utilizing comprehensions, you keep the code more readable and expressive.
- Things to Consider:
While comprehensions make your code concise, it’s essential to ensure they remain readable. For very complex transformations, traditional loops might be clearer. Also, comprehending over large datasets can consume more memory as the entire list is generated in memory, unlike with generators.
Comprehensions in Python offer a compact and elegant way to define collections. They are a hallmark of Python’s commitment to readability and the principle that “There should be one– and preferably only one –obvious way to do it.”
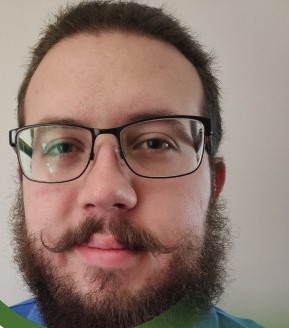
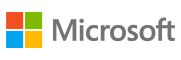