How to implement logging in Python?
Logging is a fundamental aspect of software development, providing a way to record and diagnose application behaviors and potential issues. Here’s a concise overview of how to employ logging in Python:
- Built-in `logging` Module:
Python’s standard library includes the `logging` module, which offers flexible logging of messages to different outputs and at varying severity levels.
- Basic Logging:
The simplest way to start logging is by using the basic configuration provided by the module:
```python import logging logging.basicConfig(level=logging.INFO) logging.info('This is an info message') ```
The `basicConfig` function sets up the default handler, so logs will be displayed to the console.
- Log Levels:
The logging module defines a set of severity levels for messages:
– DEBUG: Detailed information, typically of interest only when diagnosing problems.
– INFO: Used to confirm that things are working as expected.
– WARNING: An indication that something unexpected happened, or there might be a problem in the near future.
– ERROR: Due to a more serious problem, the software hasn’t been able to perform some function.
– CRITICAL: A very serious error, indicating the program itself may be unable to continue running.
- Logging to a File:
Instead of displaying logs to the console, you can direct them to a file:
```python logging.basicConfig(filename='app.log', level=logging.INFO) ```
- Advanced Logging:
– Formatters: Define a specific format for the log messages.
– Handlers: Control where your log messages go. By default, it’s the console, but it can be a file, HTTP server, or other outputs.
– Filters: Provide finer-grained control over which log records are output.
- Logger Objects:
While the module provides a default logger, you can create customized logger objects, especially useful when developing libraries or working with larger codebases. This allows different parts of an application or different libraries to log independently of each other.
- Exception Logging:
Use the `exception` method of the logger to capture and log exception information, very handy for debugging.
- Integration with Third-party Services:
Many services (like Sentry, Loggly, etc.) provide libraries or handlers to integrate with Python’s logging system, allowing for advanced logging analytics and monitoring.
Python’s `logging` module provides a powerful and flexible framework for capturing a wide range of messages in different formats and outputs. Properly implemented logging is invaluable for monitoring, debugging, and maintaining applications in production and development environments alike.
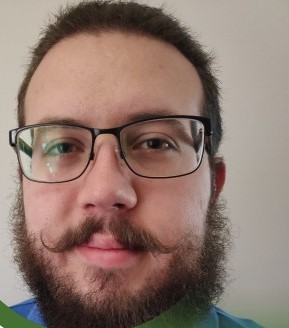
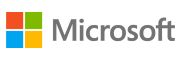