How to make a request to a website using Python?
Making requests to websites or web services is a fundamental operation for many applications, especially in the age of cloud computing and APIs. In Python, one of the most popular and user-friendly libraries to facilitate this is `requests`.
Using the `requests` Library:
- Installation:
To begin, you need to install the `requests` library. This can be done easily using pip:
``` pip install requests ```
- Making a Basic GET Request:
The most common operation is to fetch data from a website or API endpoint using a GET request.
```python import requests response = requests.get('https://www.example.com') # Check if the request was successful if response.status_code == 200: content = response.text # or response.json() if dealing with a JSON response ```
- POST Requests:
If you need to send data to an endpoint, for instance, when submitting form data or interacting with an API, you’d use a POST request.
```python data = {'key': 'value', 'name': 'Alice'} response = requests.post('https://www.api.com/endpoint', data=data) ```
- Handling Query Parameters:
If you’re interacting with an API or a website that requires query parameters, `requests` makes it straightforward.
```python params = {'query': 'Python', 'page': 2} response = requests.get('https://www.search.com/', params=params) ```
- Headers and Authentication:
Many APIs require custom headers or authentication. The `requests` library provides intuitive mechanisms for these:
```python headers = {'User-Agent': 'my-app/0.0.1'} auth = ('username', 'password') # Basic authentication response = requests.get('https://www.api.com/secure', headers=headers, auth=auth) ```
- Error Handling:
It’s essential to handle potential errors gracefully, such as timeouts or unreachable URLs. Using `response.raise_for_status()` can help identify and raise HTTP errors as exceptions.
Python, with the `requests` library, offers a streamlined way to interact with websites and web services. Its simplicity and rich feature set make it the go-to library for web requests in Python, allowing developers to fetch or send data to web resources with minimal hassle. Whether you’re scraping websites, interacting with RESTful APIs, or building integrations, understanding how to make web requests in Python is indispensable.
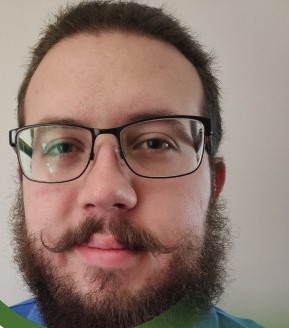
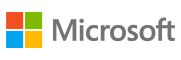