What are Python’s memory management and garbage collection mechanisms?
Python’s memory management and garbage collection are crucial components that ensure efficient use of system resources, allowing programs to run smoothly. Here’s a succinct explanation:
- Automatic Memory Management:
Python handles memory allocation automatically. When you create an object, Python’s memory manager allocates memory from the private heap specific to the Python process. The allocation ensures that developers don’t have to manage memory manually, reducing the risk of issues like memory leaks.
- Reference Counting:
At the heart of Python’s memory management is reference counting. Every object has an associated reference count, which is incremented when a reference to the object is stored somewhere (like in a variable) and decremented when a reference goes out of scope or is deleted. When the reference count of an object drops to zero, indicating that it’s no longer accessible, its memory is reclaimed.
- Garbage Collection:
While reference counting is effective, it has limitations. Circular references, where two or more objects refer to each other, prevent their reference counts from ever dropping to zero, even if they’re no longer accessible from the main program. To handle such cases, Python incorporates a garbage collector, which specifically deals with circular references.
The garbage collector works based on generational garbage collection. Objects are categorized into three generations (Gen0, Gen1, and Gen2). New objects start in Gen0, and as they survive garbage collection cycles, they move to higher generations. Since young objects (in Gen0) tend to become unreachable more quickly, the collector runs more frequently on lower generations, optimizing performance by not frequently checking long-lived objects in higher generations.
- Memory Pools and Blocks:
To further optimize memory allocation and reuse, Python’s memory manager uses a system of memory pools and blocks. Objects of similar sizes are grouped together in blocks within a pool, ensuring efficient memory usage and minimizing fragmentation.
- Manual Intervention:
Although Python handles memory management automatically, developers can influence it. Using the `gc` module, one can manually run the garbage collector or disable it for optimization during time-critical sections of code.
Python’s memory management combines automatic memory allocation, reference counting, and a generational garbage collector to manage system resources efficiently. These mechanisms work behind the scenes, ensuring that developers can focus on writing code without being bogged down by intricate memory details.
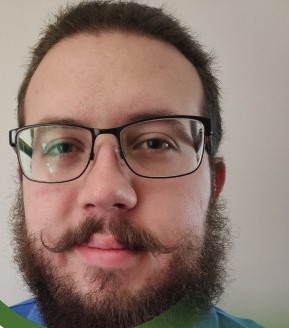
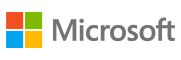