How to check the memory usage of my Python program?
Monitoring memory usage is essential for optimizing performance and ensuring that your Python program runs efficiently. Here’s a clear overview of how to gauge memory consumption in a Python application:
- Built-in `sys` Module:
– Python’s standard library provides the `sys` module, which includes a `getsizeof` function. By using `sys.getsizeof(object)`, you can obtain the memory size of the given object in bytes. However, remember that this method gives the immediate size of an object and doesn’t account for referenced objects.
- Third-party Tool: `psutil`:
– The `psutil` library allows developers to retrieve information on system utilization (CPU, memory, disks, etc.). After installing it (`pip install psutil`), you can use `psutil.Process(os.getpid()).memory_info()` to get detailed memory usage of the current Python process.
- Using `memory-profiler`:
– The `memory-profiler` package is an excellent tool for line-by-line analysis of memory usage for Python scripts. Install it using `pip install memory-profiler`.
– To profile a script, you’d add a `@profile` decorator to the functions you wish to inspect. Running the script with the `mprof` command (provided by `memory-profiler`) will then provide a detailed report of memory usage line-by-line.
– Additionally, `mprof` can generate plots showing memory consumption over time.
- `tracemalloc` Module:
– Available in Python 3.4 and above, `tracemalloc` is a built-in module that provides insights into the source of memory allocations. Once enabled, it can trace where memory blocks were allocated, allowing you to pinpoint which parts of your code consume the most memory.
- Monitoring At OS Level:
– Operating systems have built-in tools to monitor processes’ memory usage. Tools like `top` and `htop` on Linux, Task Manager on Windows, or Activity Monitor on macOS can be used to observe the memory footprint of running Python processes.
- Jupyter Notebook:
– If you’re using Jupyter Notebook, the `%memit` magic command (from the `memory-profiler` extension) can show memory usage for individual operations, which is handy for interactive development.
Keeping tabs on your Python program’s memory usage is crucial for optimization and ensuring efficient resource utilization. Several built-in and third-party tools make this task straightforward, giving developers insights into how memory is being consumed and highlighting areas that might require optimization.
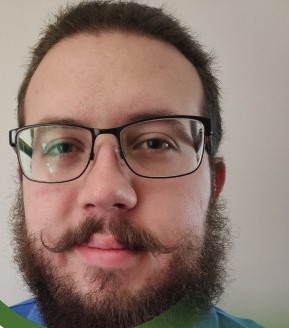
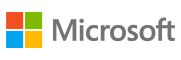