What is monkey patching in Python?
Monkey patching is a programming technique where you modify or extend existing classes or modules at runtime. This means changing a module or class’s behavior without directly altering its source code. In Python, due to its dynamic nature, monkey patching is straightforward and can be immensely powerful, but it comes with caveats.
- Usage Scenarios:
Monkey patching can be used for a variety of purposes:
– Debugging and Diagnostics: Injecting debugging logic into existing functions or methods to trace issues.
– Ad-hoc Fixes: If you’re using a third-party library and discovered a bug, rather than waiting for an official fix, you can use monkey patching as a temporary solution.
– Enhancing: Adding new methods or properties to existing classes, especially when subclassing isn’t an option.
- How It’s Done:
Because everything in Python (including functions and methods) is an object and can be re-assigned, you can easily replace a method or function with another:
```python def new_method(self): return "New Behavior" SomeClass.some_method = new_method ```
After the assignment, any call to `SomeClass.some_method` will use `new_method`.
- Caveats and Considerations:
– Maintenance Challenges: Overusing monkey patching can make your codebase difficult to understand and maintain. Future developers, including your future self, might get confused when the behavior of external libraries doesn’t match their documentation.
– Compatibility Issues: If the original library or module gets updated, your monkey patches might break if they rely on internal behavior or structures that have changed.
– Ordering Matters: The order in which monkey patches are applied is crucial, especially when multiple patches target the same functionality.
- Recommendations:
Monkey patching should be used judiciously. It’s a powerful tool but can easily be misused. Always document your patches clearly and, if possible, keep them isolated in dedicated modules or files to ensure clarity. Whenever you use monkey patching as a temporary solution, set a plan to replace the patch with a more permanent solution, such as adopting a library’s official fix or switching to a better-maintained library.
Monkey patching in Python allows dynamic alterations to modules and classes, offering flexibility in debugging, extending, or fixing third-party code. However, the technique requires careful consideration to ensure the long-term health and clarity of a project.
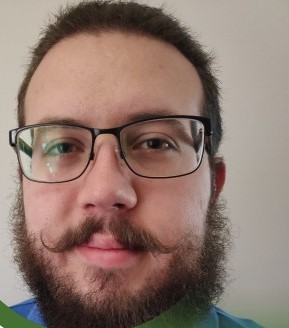
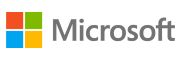