How to use multi-threading or multi-processing in Python?
Leveraging multi-threading or multi-processing is essential when looking to improve the performance of certain tasks or make better use of system resources. Here’s an overview of how to harness these in Python:
- Multi-threading with the `threading` Module:
– Overview: Threads are the smallest unit of a process that can be executed independently. Multi-threading involves several threads running within the same process, sharing the same memory space.
– Usage: The `threading` module provides tools to create and manage threads. To use it, you define a function that you wish to run as a separate thread, and then create a new `Thread` instance with this function as the target. Call the `start()` method on the thread object to initiate it.
– Consideration: Due to Python’s Global Interpreter Lock (GIL), native threads cannot execute Python bytecode in true parallel. Thus, for CPU-bound tasks, multi-threading may not offer a performance boost. However, it’s beneficial for I/O-bound tasks like network or disk operations.
- Multi-processing with the `multiprocessing` Module:
– Overview: Multi-processing involves running tasks in separate memory spaces and separate processes. This approach bypasses the GIL, allowing for true parallel execution, especially beneficial for CPU-bound tasks.
– Usage: The `multiprocessing` module provides a `Process` class similar to the `threading` module’s `Thread` class. Define your target function and create `Process` objects. Call `start()` on them to execute. There’s also a `Pool` class to parallelize the execution of a function across multiple input values.
– Consideration: Since each process runs in its own memory space, the overhead is higher than threading, but it ensures no memory conflicts or contention issues.
- Choosing Between Them:
– For I/O-bound tasks (like file operations or network requests), multi-threading could be a better choice due to its lower overhead.
– For CPU-bound tasks (like heavy computation), multi-processing is typically more effective because it bypasses the GIL and leverages multiple CPU cores.
Python provides robust tools for both multi-threading and multi-processing. The choice between them depends on the nature of the task and the specific challenges you’re looking to address. Properly used, these tools can significantly enhance the performance and responsiveness of Python programs.
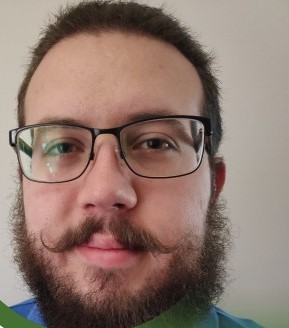
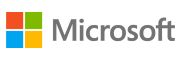