How to make Python code run faster?
Optimizing Python code is a multifaceted endeavor. Here’s a concise guide to techniques that can make your Python code run faster:
- Profiling Before Optimizing:
Before diving into optimization, determine where the bottlenecks are. Use tools like `cProfile` or `timeit` to measure execution times and identify the slowest parts of your code.
- Efficient Data Structures:
Often, a significant speed-up can be achieved by simply using the right data structure. For instance, using `set` for membership testing is faster than using a `list`.
- Built-in Functions and Libraries:
Make the most of Python’s standard library. Built-in functions and modules like `itertools`, `collections`, and `functools` are optimized and often faster than custom-made alternatives.
- Algorithmic Improvements:
Assess the algorithms you’re using. A more efficient algorithm can reduce computation time exponentially. For example, using a sorting algorithm with O(n log n) complexity instead of O(n^2) can make a world of difference for large datasets.
- Just-in-time Compilation:
Tools like Numba can compile Python code just-in-time, making certain functions run at near-C speed.
- C Extensions:
Critical code sections can be rewritten in C or C++ and then integrated into Python using tools like `Cython` or `SWIG`.
- Parallelization:
For tasks that can be broken down and run simultaneously, using multi-threading (with `threading` or `concurrent.futures`) or multi-processing (`multiprocessing` module) can lead to significant speed-ups. However, be aware of Python’s Global Interpreter Lock (GIL) when using threads.
- External Libraries:
Some optimized libraries can speed up specific tasks. For instance, for numerical operations, `NumPy` is significantly faster than native Python due to its underlying C implementation.
- Avoiding Global Variables:
Access to global variables is slower than local variables. Where possible, use local variables.
- Lazy Evaluation:
Using generators (`yield` keyword) can help by processing data on-the-fly and using memory more efficiently, especially with large datasets.
- Memory and Caching:
Techniques like memoization can avoid repeated calculations. The `functools.lru_cache` decorator can be handy for this.
While Python might not be as fast as compiled languages like C or Java out of the box, various techniques and tools can be employed to speed up your Python code. Always profile first to identify bottlenecks and then apply the most appropriate optimization strategy.
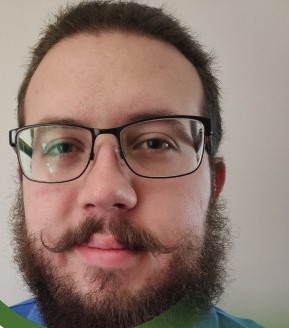
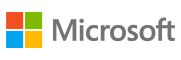