How to Use Python Functions to Parse JSON Data
In this digital age, data is our most valuable asset. With an ever-increasing volume of data comes the necessity to understand, interpret and extract meaningful information from it.
JavaScript Object Notation (JSON) has emerged as a widely adopted standard for data exchange due to its lightweight nature and easy-to-read format. When dealing with JSON data, Python – a high-level, versatile programming language – offers a variety of methods for parsing, navigating, and manipulating this data. This blog post will dive deep into how to utilize Python functions to parse JSON data effectively.
Understanding JSON
Before we dive into Python’s role, let’s briefly touch upon what JSON is. JSON is a text-based data format following JavaScript object syntax, frequently used to transmit data over a network, typically between a server and a web application. JSON objects contain data in key-value pairs and can include nested JSON objects, arrays, booleans, numbers, strings, and null.
Working With JSON in Python
Python’s built-in json module transforms JSON data into Python data structures and vice versa, also known as serialization and deserialization. Here is how you import the json module in
python import json
JSON to Python
Let’s start by converting JSON data into Python data types, a process known as deserialization. The json module provides two methods for this: json.load() and json.loads().
– json.load(): This method parses JSON data from a file.
– json.loads(): This method parses JSON data from a string.
Here’s an example of json.loads():
import json json_string = '{"name": "John", "age": 30, "city": "New York"}' python_dict = json.loads(json_string) print(python_dict)
Output:
{'name': 'John', 'age': 30, 'city': 'New York'}
Python to JSON
Now, let’s convert Python data types into JSON, a process known as serialization. For this, the json module provides json.dump() and json.dumps().
– json.dump(): This method writes Python data to a file in JSON format.
– json.dumps(): This method writes Python data to a JSON formatted string.
Here’s an example of json.dumps():
import json python_dict = {'name': 'John', 'age': 30, 'city': 'New York'} json_string = json.dumps(python_dict) print(json_string)
Output:
'{"name": "John", "age": 30, "city": "New York"}'
Formatting JSON Output
The json.dumps() method has parameters to make the result more readable. The parameters indent, separators, and sort_keys can make your JSON data more readable, organized, and easier to understand.
import json python_dict = {'name': 'John', 'age': 30, 'city': 'New York'} json_string = json.dumps(python_dict, indent=4, separators=(". ", " = "), sort_keys=True) print(json_string)
Output:
{ "age = 30. " "city = New York. " "name = John. " }
Handling Complex Python Objects
While the json module can serialize and deserialize basic Python data types, it struggles with complex Python objects like a custom class instance. You can resolve this by defining your methods or using libraries like ‘jsonpickle’.
Working with JSON Arrays
A JSON array is an ordered collection of values, similar to a list in Python. You can easily parse JSON arrays using the json.loads() or json.load() functions.
import json json_array = '[{"name": "John", "age": 30, "city": "New York"},{"name": "Jane", "age": 28, "city": "Chicago"}]' python_list = json.loads(json_array) print(python_list)
Output:
[{'name': 'John', 'age': 30, 'city': 'New York'}, {'name': 'Jane', 'age': 28, 'city': 'Chicago'}]
Navigating and Extracting Data
Once you’ve converted JSON data to a Python data structure, you can manipulate it using standard Python operations. For instance, you could iterate over a list of dictionaries returned from JSON data like so:
for person in python_list: print(person['name'])
Output:
John Jane
This example prints out the name of each person in the list.
Conclusion
Python’s built-in json module makes it easy to parse JSON data and transform it into Python data structures. Whether you’re dealing with simple or complex data structures, JSON arrays, or even nested JSON data, Python provides a flexible and efficient approach to handle JSON data. Understanding how to parse and work with JSON data is a vital skill in the current data-driven world, and Python is an excellent tool for this task. As we continue to generate more data, these skills will become increasingly valuable.
Table of Contents
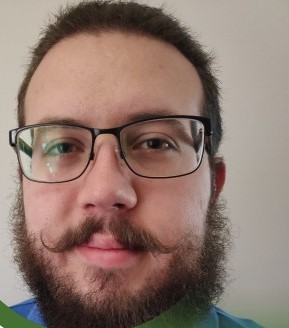
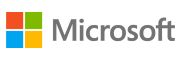