How to parse XML and HTML in Python?
Parsing XML and HTML in Python is an essential task when working with data obtained from the web or other structured documents. Here’s how you can approach this:
- XML Parsing:
ElementTree (stdlib):
Python’s standard library provides the `xml.etree.ElementTree` module for XML parsing. It allows you to read XML documents, navigate the XML tree, and extract data from elements and attributes.
Example:
```python import xml.etree.ElementTree as ET root = ET.parse('filename.xml').getroot() for child in root: print(child.tag, child.attrib) ```
- HTML Parsing:
Beautiful Soup:
One of the most popular libraries for HTML (and XML) parsing, Beautiful Soup transforms a complex HTML document into a tree of Python objects, such as tags, navigable strings, and comments. It provides easy-to-use methods and Pythonic idioms for iterating and searching the parse tree.
Example:
```python from bs4 import BeautifulSoup soup = BeautifulSoup(html_content, 'html.parser') for link in soup.find_all('a'): print(link.get('href')) ```
lxml:
A library that provides a fast yet flexible tool for parsing XML and HTML. It combines the speed and XML compatibility of `libxml2` with the ease of use of ElementTree.
Example:
```python from lxml import html tree = html.fromstring(html_content) links = tree.xpath('//a/@href') ```
- Tips and Warnings:
– While parsing HTML, always use a parser designed for HTML and not XML since HTML is often not well-formed.
– For web scraping tasks where you need to parse HTML from web pages, it’s courteous and recommended to check a website’s `robots.txt` file to ensure you’re allowed to scrape and access the data.
Python offers multiple robust tools for parsing XML and HTML. The choice between them usually hinges on the specific needs of the project and the personal preferences of the developer.
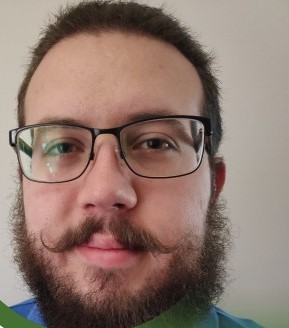
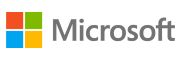