How to profile my Python code for performance?
Profiling is an essential technique for identifying performance bottlenecks in code. For Python developers, several tools and methodologies are available to profile and thereby optimize their code:
- The `cProfile` Module:
The built-in `cProfile` module is a commonly used profiler for Python programs. It provides a way to see how often and for how long each function is called, making it easier to identify bottlenecks.
– Usage: Simply run your script with `cProfile`, like this: `python -m cProfile your_script.py`. This will print a table of statistics, sorted by the internal time taken by each function.
- Visualizing Profiles with `pyprof2calltree`:
While `cProfile` provides detailed stats, visualizing these stats can be more insightful. Using `pyprof2calltree` in combination with tools like `kcachegrind` or `qcachegrind` allows you to see a visual representation of the profiling data.
- The `timeit` Module:
For small code snippets where you want to assess performance, `timeit` is the perfect tool. It provides a simple way to time small bits of Python code, ensuring accuracy by running the code multiple times and averaging the results.
- Line Profilers:
While `cProfile` gives a function-by-function breakdown, sometimes you need a line-by-line analysis. The `line_profiler` tool provides this capability. After installing it, you can use the `@profile` decorator to the functions you want to profile, and then run the script with `kernprof`.
- Memory Profiling:
If you suspect that your performance issues are related to memory usage rather than CPU time, tools like `memory_profiler` can help. It provides line-by-line memory consumption for Python scripts, which can be crucial for identifying memory leaks or inefficient data structures.
- Profiling in Integrated Development Environments (IDEs):
Many modern IDEs, like PyCharm or Visual Studio Code, come with integrated profiling tools. These can be particularly user-friendly and offer rich interfaces to explore the profiling data.
Profiling is a vital step in optimizing Python code. By using the above tools, developers can gain insights into the performance characteristics of their programs, enabling them to make informed decisions about where to focus their optimization efforts. As always, it’s essential to profile actual use-case scenarios to ensure that optimization efforts target real-world performance bottlenecks.
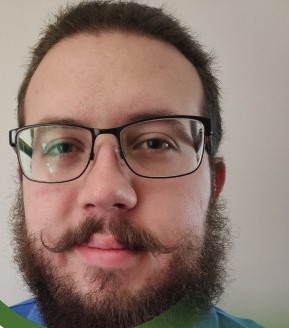
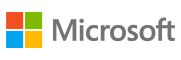