How to plot data in Python?
Python offers a variety of libraries for plotting and visualizing data, but the most renowned and widely used among them is Matplotlib. It provides a comprehensive platform for creating static, animated, and interactive visualizations.
Plotting Data using Matplotlib:
- Installation:
Before you can use Matplotlib, you must install it. The most common way to do this is via pip:
``` pip install matplotlib ```
- Basic Plotting:
At its core, Matplotlib provides a simple way to plot data. Here’s a brief overview:
```python import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y = [1, 4, 9, 16, 25] # Create a figure and axis plt.plot(x, y) # Add a title and labels plt.title("Basic Plot") plt.xlabel("X-axis") plt.ylabel("Y-axis") # Display the plot plt.show() ```
- Different Types of Plots:
Matplotlib supports a wide variety of plots, including:
– Line plots
– Bar charts
– Histograms
– Scatter plots
– Pie charts
… and many more. You choose the type of plot based on the nature of your data and the insights you want to derive.
- Styling & Customization:
One of the strengths of Matplotlib is its flexibility. You can customize almost every aspect of a plot, including its size, colors, legends, and annotations. The library provides default styles, but you have the power to adjust them as needed.
- Integration with Pandas:
If you’re working with dataframes using the Pandas library, Matplotlib integrates seamlessly. Pandas data structures have built-in methods for visualization, making it easy to plot data directly from a dataframe or series.
- Other Libraries:
While Matplotlib is powerful, other libraries like Seaborn (built on top of Matplotlib) offer more aesthetically pleasing and complex visualizations with less boilerplate code.
Matplotlib provides a robust foundation for visualizing data in Python. With its flexibility and extensive functionality, you can craft compelling plots and charts tailored to your data. Whether you’re doing exploratory data analysis, presenting results, or building interactive applications, understanding how to use Python’s plotting capabilities is a valuable skill.
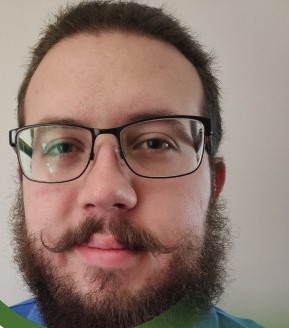
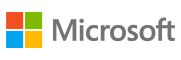