How to process images in Python?
Processing images with Python has become a standard task thanks to a wealth of libraries tailored for this purpose. One of the most renowned and comprehensive libraries for this job is Pillow, which is an improved fork of the Python Imaging Library (PIL).
- Installation:
Before starting, you’ll need to install the library:
```bash pip install Pillow ```
- Basic Operations:
With Pillow, you can perform numerous image operations:
– Loading and Saving: To open an image, use the `Image` class. Saving an image in a different format is as simple as specifying a new extension.
```python from PIL import Image img = Image.open('path_to_image.jpg') img.save('new_path.png') ```
– Cropping, Rotating, and Resizing: These are fundamental operations that can be performed effortlessly.
```python cropped = img.crop((left, upper, right, lower)) rotated = img.rotate(90) # Rotate by 90 degrees resized = img.resize((width, height)) ```
– Filtering and Effects: Pillow comes with pre-built filters and effects to enhance images.
```python from PIL import ImageFilter blurred = img.filter(ImageFilter.BLUR) ```
- Advanced Tasks:
– Drawing on Images: Using the `ImageDraw` module, you can create graphics, write text, or draw shapes directly onto your images.
– Color Manipulations: Convert images to grayscale, adjust brightness, or enhance color with the `ImageEnhance` module.
- Other Libraries:
For more advanced image processing or computer vision tasks, **OpenCV** is a robust choice. It offers features like object detection, facial recognition, and more. If you’re looking into deep learning-related image tasks, **TensorFlow** and **PyTorch** offer comprehensive tools and integrations for image-based neural networks.
Python, with the help of libraries like Pillow and OpenCV, provides a powerful suite of tools for both basic and advanced image processing. Whether you’re building an application that handles photos or delving into the world of computer vision, Python has got you covered.
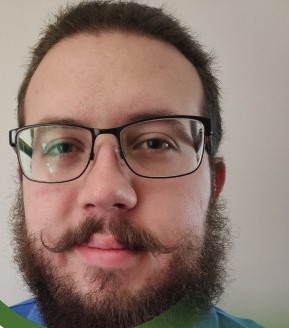
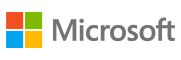