How to use the `range` function in Python?
The `range` function in Python is a versatile utility that generates a sequence of numbers. It’s commonly employed in loops and other places where an iterable is needed. The numbers produced by `range` are not stored as a list in memory, making it memory-efficient. Instead, the numbers are generated on-the-fly, one by one, as you iterate over them.
Usage:
The `range` function can be called using one, two, or three arguments:
- Single Argument: When provided with a single argument `n`, `range` produces numbers starting from 0 and going up to, but not including, `n`.
– Example: `range(3)` produces the sequence `0, 1, 2`.
- Two Arguments: With two arguments, `start` and `stop`, `range` starts the sequence at `start` and goes up to, but not including, `stop`.
– Example: `range(2, 5)` produces the sequence `2, 3, 4`.
- Three Arguments: When given three arguments, `start`, `stop`, and `step`, `range` starts at `start`, increments by `step`, and goes up to, but doesn’t include, `stop`. The `step` can also be negative, allowing for counting downwards.
– Example: `range(2, 10, 2)` produces the sequence `2, 4, 6, 8`.
– Example with negative step: `range(10, 2, -2)` produces the sequence `10, 8, 6, 4`.
Common Use Cases:
– For Loops: `range` is frequently used in `for` loops to repeat an action a specified number of times.
– List Generation: Although `range` itself produces a generator-like sequence, it can be used in combination with the `list` constructor to generate a list of numbers.
– Iterative Tasks: Any task where you need to step through a sequence of numbers, whether it’s indexing an iterable or running a repetitive calculation, `range` is your go-to function.
The `range` function is an invaluable tool in Python for creating number sequences efficiently. It offers flexibility in defining the start, stop, and step of the sequence, catering to a wide array of programming scenarios. Familiarity with its usage is essential for anyone delving into Python’s iterative and looping constructs.
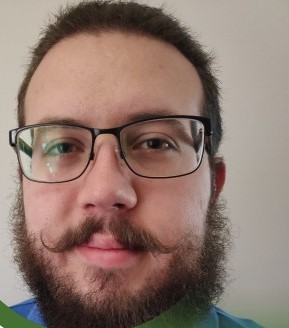
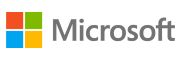