How to read and write files in Python?
Reading and writing files is a fundamental operation in many applications, and Python offers intuitive mechanisms for file handling.
Opening a File:
To work with a file, you first need to open it using the built-in `open` function. This function returns a file object and takes two main arguments: the file path and the mode (`’r’` for reading, `’w’` for writing, `’a’` for appending, and `’b’` to read/write in binary mode).
Reading from a File:
Once a file is opened in read mode (`’r’`), you can use the `read()` method to read its contents. For reading line-by-line, methods like `readline()` or `readlines()` are available. Iterating over the file object directly also yields its lines, making it memory-efficient for large files.
Writing to a File:
To write to a file, you’d open it in write (`’w’`) or append (`’a’`) mode. The `write()` method of the file object can then be used to write data. Note that in write mode, the file content is overwritten, while in append mode, new data is added to the end.
Closing a File:
It’s crucial to close a file after you’re done with it to free up system resources. You do this using the `close()` method. However, it’s easy to forget to close files, which can lead to issues.
To ensure files are properly closed, Python offers the `with` statement, a context manager that automatically closes the file once operations within the block are completed, even if exceptions occur.
File Paths:
When specifying file paths, it’s good practice to use the `os.path` module or the newer `pathlib` module to ensure your paths are platform-independent.
Python provides simple and effective tools for reading and writing files. By understanding these mechanisms, along with best practices like always using the `with` statement for file operations, developers can confidently handle files in their applications, ensuring data integrity and efficient resource usage.
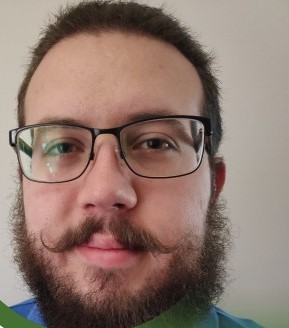
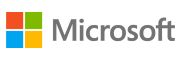