Python Tutorial: Understanding the Recursion and Iteration
When it comes to solving problems in programming, there are two fundamental techniques that every programmer should be familiar with: recursion and iteration. These techniques are used to control the flow of a program and solve complex problems by breaking them down into smaller, more manageable components. In this tutorial, we will delve into the concepts of recursion and iteration, explore their differences, advantages, and provide you with code samples to help solidify your understanding.
Table of Contents
1. Introduction to Recursion and Iteration
1.1. What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. It’s like a mathematical induction, where you break down a problem into smaller instances of the same problem until you reach a base case that can be solved directly. Recursive functions often have two components: a base case and a recursive case.
1.2. What is Iteration?
Iteration, on the other hand, involves repeating a set of instructions in a loop until a certain condition is met. It’s a fundamental programming concept that allows you to perform repetitive tasks efficiently. In Python, iteration is achieved using various looping constructs like for loops and while loops.
1.3. Why are They Important?
Recursion and iteration both play vital roles in solving problems effectively. Depending on the nature of the problem, one technique might be more suitable than the other. By understanding the differences between them, you can choose the best approach to tackle various programming challenges.
2. Understanding Recursion
2.1. How Recursion Works
Recursion starts with a problem that is divided into smaller, similar subproblems. The function is called with the smaller subproblem, and the process continues until the base case is reached. At that point, the solution for the base case is returned, and the recursion “unwinds,” combining the solutions of the subproblems to solve the original problem.
2.2. Base Case and Recursive Case
Every recursive function must have a base case, which is the simplest version of the problem that can be solved directly. Additionally, the function must have a recursive case, where the problem is divided into smaller instances and the function calls itself with those instances.
Let’s take a look at a simple example of calculating the factorial of a number using recursion:
python def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1) In this example, the base case is when n is 0, and the recursive case calculates n * factorial(n - 1).
2.3. The Call Stack
Recursion relies on a call stack to keep track of function calls. Each time a function is called, its parameters and local variables are pushed onto the stack. As the recursion “unwinds,” the stack is popped, and the program continues to execute.
2.4. Pros and Cons of Recursion
Recursion can lead to elegant and concise code for certain problems. It’s well-suited for tasks that can be broken down into smaller instances of the same problem. However, excessive recursion can lead to a deep call stack, consuming more memory and potentially causing a stack overflow error. It’s essential to strike a balance between readability and efficiency when using recursion.
3. Mastering Iteration
3.1. How Iteration Works
Iteration involves executing a set of instructions repeatedly until a specified condition is met. It’s particularly useful when the task at hand involves a fixed number of repetitions or when you want to process elements in a collection one by one.
In Python, you can use various looping constructs for iteration, including the for loop and the while loop.
3.2. Looping Constructs in Python
For Loop:
The for loop is used to iterate over a sequence (such as a list, tuple, string, etc.) and execute a block of code for each element in the sequence.
python numbers = [1, 2, 3, 4, 5] for num in numbers: print(num)
While Loop:
The while loop repeatedly executes a block of code as long as a given condition is true.
python count = 0 while count < 5: print(count) count += 1
3.3. Use Cases of Iteration
Iteration is essential for tasks like processing large datasets, traversing tree structures, and implementing algorithms that involve repetitive steps. It offers better control over memory usage compared to recursion, as the call stack is not involved.
4. Comparing Recursion and Iteration
4.1. Performance Considerations
Recursion can sometimes be less efficient due to the overhead of managing function calls and the call stack. For problems that can be solved iteratively, using recursion might lead to slower execution. Iteration, on the other hand, is often more efficient when the task can be accomplished using a loop.
4.2. Readability and Maintainability
Recursion can lead to elegant code that closely mirrors the problem’s definition. However, this elegance can come at the cost of readability, especially for complex recursive functions. Iteration, with its explicit loop structure, can sometimes be more readable and easier to maintain.
5. Code Samples and Examples
5.1. Recursive Factorial Calculation
python def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1) result = factorial(5) print(result) # Output: 120
5.2. Iterative Factorial Calculation
python def iterative_factorial(n): result = 1 for i in range(1, n + 1): result *= i return result result = iterative_factorial(5) print(result) # Output: 120
5.3. Recursive vs. Iterative Fibonacci Sequence
python def recursive_fibonacci(n): if n <= 1: return n else: return recursive_fibonacci(n - 1) + recursive_fibonacci(n - 2) def iterative_fibonacci(n): if n <= 1: return n fib_minus_2, fib_minus_1 = 0, 1 for _ in range(2, n + 1): fib = fib_minus_2 + fib_minus_1 fib_minus_2, fib_minus_1 = fib_minus_1, fib return fib_minus_1 print(recursive_fibonacci(6)) # Output: 8 print(iterative_fibonacci(6)) # Output: 8
Conclusion
Both recursion and iteration are powerful tools in a programmer’s toolkit. They each have their strengths and weaknesses, making them suitable for different scenarios. By understanding the concepts, benefits, and limitations of recursion and iteration, you can make informed decisions when designing and implementing solutions to various programming challenges. Remember that practice is key to mastering these techniques, so don’t hesitate to experiment with different problems and explore how recursion and iteration can be used effectively in your code.
Table of Contents
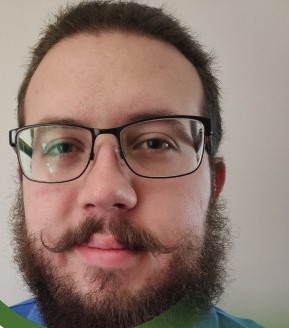
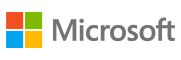