Working with Regular Expressions for Text Processing in Python
Text processing is a fundamental part of many data analysis, natural language processing, and web scraping tasks. Whether you are extracting specific information from a large dataset, validating user input, or parsing text files, regular expressions (regex) are a powerful tool that can save you time and effort. In this blog, we will explore how to leverage regular expressions in Python to efficiently manipulate and extract information from text.
1. Introduction to Regular Expressions
What are Regular Expressions?
Regular expressions are a sequence of characters that define a search pattern. They provide a concise and flexible way to match and manipulate text data. In Python, regular expressions are supported through the built-in re module, which offers a wide range of functions for working with regex.
Advantages of Using Regular Expressions
- Flexibility: Regular expressions allow you to express complex patterns in a compact and readable form.
- Pattern Matching: You can easily match and extract specific substrings from text data.
- Text Manipulation: Regular expressions can be used for find-and-replace operations and data transformation tasks.
- Validation: They are great for validating input data like emails, phone numbers, and more.
- Efficiency: When used correctly, regex can significantly speed up text processing tasks.
Python’s re Module
Python’s re module provides several functions to work with regular expressions, including re.search(), re.match(), re.findall(), re.sub(), and more. It’s essential to import the re module before using regular expressions in your Python code.
python import re
2. Basic Regular Expression Patterns
Matching Text Literals
The simplest regular expression pattern is a literal match. For example, to find the word “python” in a text, you can use the following code:
python import re text = "I love Python programming language." pattern = r"Python" match = re.search(pattern, text) if match: print("Found:", match.group()) else: print("Not Found")
The output will be:
makefile Found: Python
Character Classes
Character classes allow you to match any one of a set of characters. Commonly used character classes include:
- \d: Matches any digit (0-9).
- \D: Matches any non-digit character.
- \w: Matches any alphanumeric character (word character).
- \W: Matches any non-alphanumeric character (non-word character).
- \s: Matches any whitespace character (space, tab, newline).
- \S: Matches any non-whitespace character.
For instance, to extract all the phone numbers from a string, you can use the following pattern:
python import re text = "Contact us at +123-456-7890 or email@example.com." pattern = r"\+\d{3}-\d{3}-\d{4}" matches = re.findall(pattern, text) print(matches)
The output will be:
css ['+123-456-7890']
Metacharacters
Metacharacters are characters with a special meaning in regular expressions. Some common metacharacters include:
- .: Matches any character except a newline.
- ^: Anchors the match to the start of the string.
- $: Anchors the match to the end of the string.
- *: Matches zero or more occurrences of the preceding character.
- +: Matches one or more occurrences of the preceding character.
- ?: Matches zero or one occurrence of the preceding character.
- |: Acts like a logical OR, allowing alternatives in the pattern.
For example, to find all words starting with “python,” ignoring the case, you can use:
python import re text = "I enjoy programming in Python. pythonista for life!" pattern = r"(?i)python\w*" matches = re.findall(pattern, text) print(matches)
The output will be:
css ['Python', 'pythonista']
Quantifiers
Quantifiers define how many occurrences of a character or group are expected. Common quantifiers include:
- {n}: Matches exactly n occurrences of the preceding character.
- {n,}: Matches n or more occurrences of the preceding character.
- {n,m}: Matches between n and m occurrences of the preceding character (inclusive).
For instance, to find all words with three or more consecutive vowels in a sentence, you can use:
python import re text = "I feel great today! Go outside and enjoy the beautiful weather." pattern = r"\b\w*[aeiou]{3,}\w*\b" matches = re.findall(pattern, text, re.IGNORECASE) print(matches)
The output will be:
css ['feel', 'beautiful', 'weather']
3. Working with Groups and Capturing
Grouping Patterns
Groups are subexpressions enclosed in parentheses. They are useful when you want to apply quantifiers to multiple characters or create complex patterns.
python import re text = "John Doe: 30 years old, Jane Smith: 25 years old" pattern = r"(\w+\s\w+):\s(\d+)\syears\sold" matches = re.findall(pattern, text) print(matches)
The output will be:
css [('John Doe', '30'), ('Jane Smith', '25')]
Capturing Groups
Groups can be used to capture specific parts of a matched pattern. You can access the captured groups using the group() method on the match object.
python import re text = "Date: 2023-07-17" pattern = r"(\d{4})-(\d{2})-(\d{2})" match = re.search(pattern, text) if match: year, month, day = match.groups() print("Year:", year) print("Month:", month) print("Day:", day)
The output will be:
yaml Year: 2023 Month: 07 Day: 17
Non-capturing Groups
If you don’t need to capture a group, you can use the non-capturing syntax (?:…).
python import re text = "Version 1.2.3 released" pattern = r"Version (?:\d+)\.(?:\d+)\.(?:\d+)" match = re.search(pattern, text) if match: print("Found:", match.group()) else: print("Not Found")
The output will be:
makefile Found: Version 1.2.3
Backreferences
Backreferences allow you to reuse captured groups within the same regex pattern. This can be handy for tasks like finding duplicate words.
python import re text = "The sun sun rises in the east" pattern = r"\b(\w+)\s+\b" matches = re.findall(pattern, text, re.IGNORECASE) print(matches)
The output will be:
css ['sun']
4. Common Use Cases for Regular Expressions
Validating Email Addresses
Email validation is a typical application of regular expressions. Here’s a simple pattern to validate email addresses:
python import re def is_valid_email(email): pattern = r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$" return re.match(pattern, email) is not None email_addresses = ["john@example.com", "jane.doe@example", "invalid@.com"] for email in email_addresses: if is_valid_email(email): print(f"{email} is a valid email address.") else: print(f"{email} is not a valid email address.")
The output will be:
kotlin john@example.com is a valid email address. jane.doe@example is not a valid email address. invalid@.com is not a valid email address.
Extracting Dates and Times
Regular expressions can help extract dates and times from text, even in various formats.
python import re text = "Meeting on 2023-07-17 at 15:30 in Room A" date_pattern = r"\d{4}-\d{2}-\d{2}" time_pattern = r"\d{2}:\d{2}" date = re.search(date_pattern, text).group() time = re.search(time_pattern, text).group() print("Date:", date) print("Time:", time)
The output will be:
yaml Date: 2023-07-17 Time: 15:30
Parsing HTML and XML
Regular expressions are not the best choice for parsing complex markup languages like HTML or XML. However, they can be useful for simple cases.
python import re html = "<p>Hello, <strong>world!</strong></p>" pattern = r"<(\w+)>(.+?)<\/>" matches = re.findall(pattern, html) for tag, content in matches: print(f"Tag: {tag}, Content: {content}")
The output will be:
yaml Tag: p, Content: Hello, Tag: strong, Content: world!
5. Tips for Writing Effective Regular Expressions
Be Specific and Precise
Regular expressions can quickly become complex and hard to read. Always strive to be as specific and precise as possible in your patterns to avoid unintended matches.
Use Non-greedy Quantifiers
By default, quantifiers like * and + are greedy, meaning they will match as much as possible. Use non-greedy versions *? and +? to match as little as possible.
Optimize Performance
Large and inefficient regular expressions can significantly slow down your code. If you notice performance issues, consider breaking the pattern into smaller, simpler parts.
6. Advanced Techniques
Lookaround Assertions
Lookaround assertions are non-capturing groups that don’t consume characters but assert specific conditions without including them in the match.
python import re text = "Match this but not that" pattern = r"\b\w+(?=\sbut\b)" matches = re.findall(pattern, text) print(matches)
The output will be:
css ['this']
Conditional Expressions
You can use conditional expressions to create regex patterns that match different alternatives based on specific conditions.
python import re text = "That is awesome" pattern = r"\b(\w+)\s+(?:(?P<verb>is)|(?:are))\s+(?(verb)awesome|cool)\b" matches = re.findall(pattern, text, re.IGNORECASE) print(matches)
The output will be:
css [('That', 'awesome')]
Substitution and Replacements
Regular expressions are not just for finding matches; they are also useful for substitutions.
python import re text = "Hello, my name is John." pattern = r"John" replacement = "Mike" new_text = re.sub(pattern, replacement, text) print(new_text)
The output will be:
csharp Hello, my name is Mike.
Conclusion
Regular expressions are a powerful tool for text processing in Python. They offer flexibility and efficiency in handling complex pattern matching and manipulation tasks. Understanding the basics of regular expressions and the re module in Python opens up a world of possibilities in data analysis, natural language processing, and beyond. With this newfound knowledge, you are ready to tackle diverse text processing challenges and supercharge your Python projects!
Table of Contents
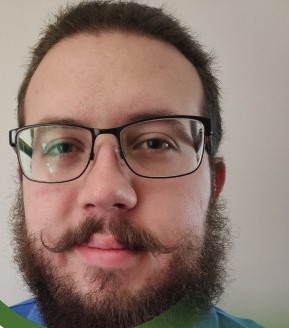
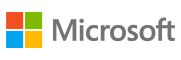