Python Tutorial: Understanding the Scope of Variables
When programming in Python, understanding the scope of variables is crucial for writing efficient and bug-free code. Variables in Python have different levels of visibility, which determine where they can be accessed and modified within your code. In this tutorial, we will delve into the intricacies of variable scopes in Python, exploring local, global, and nonlocal scopes with clear examples.
Table of Contents
1. Introduction to Variable Scopes
In programming, scope refers to the region of your code where a particular variable is accessible. Python follows a set of rules to determine which variables can be accessed from where, ensuring code integrity and preventing naming conflicts. There are three main types of variable scopes in Python:
- Local Scope: The scope within a function where variables are only accessible within that function.
- Global Scope: The outermost scope of your program, where variables are accessible throughout the entire program.
- Nonlocal Scope: An intermediate scope that allows variables to be accessed in nested functions.
Let’s explore each scope type in detail.
2. Local Scope
2.1. Defining Variables in Local Scope
When you define a variable within a function, it is said to have a local scope. This means the variable is only accessible within that function and cannot be directly accessed from outside. This is a fundamental concept of encapsulation in programming, where variables are confined to the scope where they are needed. Let’s look at an example:
python def calculate_square(number): square = number ** 2 return square result = calculate_square(5) print(result) # Output: 25 # print(square) # This would result in an error
In the example above, the variable square is defined within the calculate_square function. Trying to access square outside the function would result in an error.
2.2. Accessing Local Variables
Local variables can only be accessed within the function where they are defined. This helps prevent unintended modifications or conflicts with variables from other parts of the code. Consider this example:
python def multiply(a, b): result = a * b return result product = multiply(3, 4) # print(result) # This would result in an error
Here, the variable result is confined to the multiply function and cannot be accessed from outside.
3. Global Scope
3.1. Creating Global Variables
Variables defined outside of any function or block of code have a global scope. This means they can be accessed from any part of the program, including within functions. Global variables are often used for values that need to be shared across multiple functions or modules. Let’s look at an example:
python global_var = 10 def print_global(): print(global_var) print_global() # Output: 10
In this example, global_var is accessible within the print_global function without any issues.
3.2. Modifying Global Variables
While global variables can be accessed from within functions, modifying them requires some extra attention. If you want to modify a global variable’s value within a function, you need to use the global keyword to indicate your intention. Otherwise, Python will create a new local variable with the same name instead. Here’s an example:
python count = 0 def increment(): global count count += 1 increment() print(count) # Output: 1
By using the global keyword, you explicitly tell Python that you want to modify the global variable count.
4. Nonlocal Scope
4.1. Using the nonlocal Keyword
The nonlocal keyword is used within nested functions to indicate that a variable being accessed or modified belongs to an outer (but not global) scope. This is particularly useful when dealing with nested functions that share variables. Consider the following example:
python def outer_function(): x = 10 def inner_function(): nonlocal x x += 5 print(x) inner_function() outer_function() # Output: 15
In this example, the nonlocal keyword allows the inner_function to modify the x variable from its outer scope (outer_function), instead of creating a new local variable.
5. Scope Hierarchy
Understanding the scope hierarchy is essential to prevent naming conflicts and to ensure that your code behaves as expected. The order in which Python searches for variables is as follows:
- Local Scope: Python first searches for the variable within the current function’s scope.
- Enclosing Functions: If the variable is not found in the local scope, Python searches in the scopes of enclosing functions (if any).
- Global Scope: If the variable is still not found, Python looks in the global scope.
- Built-in Scope: Finally, if the variable is not found in any of the above scopes, Python searches in the built-in scope, which contains functions and objects provided by Python itself.
6. Best Practices
To write clean and maintainable code, consider the following best practices when working with variable scopes in Python:
- Avoid Global Variables: While global variables are useful, excessive use can lead to confusion and bugs. Whenever possible, use local variables or function parameters to avoid unexpected changes to global state.
- Limit Scope: Define variables in the smallest applicable scope. This reduces the chance of naming conflicts and improves code readability.
- Use Descriptive Names: Choose meaningful names for your variables. This makes it easier to understand their purpose and scope.
- Avoid Shadowing: Avoid naming local variables the same as variables in higher scopes. This can lead to confusion and unexpected behavior.
Conclusion
Understanding variable scopes is essential for writing efficient and maintainable Python code. By defining variables within the appropriate scope, you can prevent naming conflicts, improve code readability, and create more robust programs. Remember the distinctions between local, global, and nonlocal scopes, and apply the best practices mentioned in this tutorial to elevate your Python programming skills. Happy coding!
Table of Contents
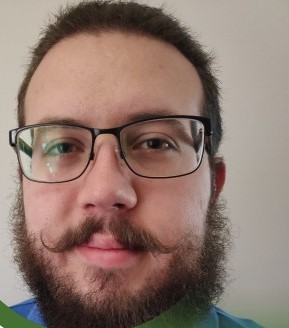
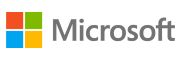