How to Use Python Functions for Scraping Twitter Data
In the digital age, data is king, and social media platforms like Twitter are treasure troves of valuable information. Whether you’re a researcher, analyst, or simply curious about trends, scraping Twitter data can provide you with valuable insights. Python, with its versatile libraries, is an ideal choice for this task. In this guide, we’ll dive into the world of scraping Twitter data using Python functions, providing you with step-by-step instructions, code samples, and best practices.
Table of Contents
1. Introduction to Twitter Data Scraping
1.1. Understanding the Importance of Data Scraping
In today’s data-driven world, information is a valuable resource. Data scraping, the process of extracting information from websites and online platforms, has become a pivotal tool for researchers, marketers, and analysts. Twitter, as a microblogging platform, is rich with real-time conversations, trends, and user interactions. By scraping Twitter data, you can uncover patterns, sentiments, and insights that can influence decision-making.
1.2. Twitter as a Data Source
Twitter hosts a wealth of data that can be valuable for various purposes:
- Social Media Analysis: Understand public sentiment towards a product, brand, or event.
- Trend Prediction: Identify emerging trends and popular topics.
- User Behavior Study: Analyze how users interact with each other and with content.
- Influencer Identification: Find key influencers in specific domains.
2. Setting Up Your Environment
2.1. Installing Python and Required Libraries
Before diving into Twitter data scraping, ensure you have Python installed. You’ll also need several libraries, with Tweepy being the star of the show. Tweepy is a Python library that simplifies the interaction with the Twitter API.
You can install Tweepy using pip:
bash pip install tweepy
2.2. Creating a Twitter Developer Account
To access Twitter’s API, you need a Twitter Developer Account. Follow these steps to set it up:
- Create a Twitter Account: If you don’t have a Twitter account, create one.
- Apply for Developer Access: Go to the Twitter Developer Platform and apply for a developer account.
- Create an App: Once your developer account is approved, create a new app. This will provide you with the necessary API keys and tokens for authentication.
3. Exploring the Tweepy Library
3.1. Installing Tweepy
With your environment ready, let’s explore the Tweepy library. Tweepy simplifies the process of accessing Twitter’s API and handling the data it returns. Here’s a simple example of how to authenticate with Tweepy using your API keys:
python import tweepy # Add your API keys and tokens here consumer_key = 'your_consumer_key' consumer_secret = 'your_consumer_secret' access_token = 'your_access_token' access_token_secret = 'your_access_token_secret' # Authenticate with Twitter's API auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) # Create an API object api = tweepy.API(auth)
This code sets up the authentication and creates an API object that you can use to interact with Twitter’s API.
4. Building Python Functions for Twitter Data Scraping
Now that you have Tweepy up and running, it’s time to build Python functions for scraping Twitter data. Let’s explore three essential functions.
Function 1: Retrieving User Information
Fetching user information is often the starting point. You can gather details like the user’s bio, follower count, and more. Here’s a function to retrieve user information using Tweepy:
python def get_user_info(username): try: user = api.get_user(screen_name=username) return { 'Username': user.screen_name, 'Name': user.name, 'Bio': user.description, 'Followers': user.followers_count, 'Following': user.friends_count, 'Tweets Count': user.statuses_count } except tweepy.TweepError as e: return {'Error': str(e)}
This function takes a username as input and returns a dictionary with relevant user information.
Function 2: Scraping User Tweets
Fetching a user’s tweets is a common task in data scraping. You can analyze their content, engagement, and trends. Here’s a function to scrape a user’s recent tweets:
python def get_user_tweets(username, count=10): try: tweets = [] for tweet in tweepy.Cursor(api.user_timeline, screen_name=username, tweet_mode='extended').items(count): tweets.append({ 'Date': tweet.created_at, 'Text': tweet.full_text, 'Likes': tweet.favorite_count, 'Retweets': tweet.retweet_count }) return tweets except tweepy.TweepError as e: return {'Error': str(e)}
This function takes a username and a count of tweets to retrieve, returning a list of dictionaries containing tweet details.
Function 3: Monitoring Hashtags
Tracking hashtags can help you gauge the popularity of a topic. Here’s a function to monitor tweets containing a specific hashtag:
python def get_hashtag_tweets(hashtag, count=10): try: tweets = [] for tweet in tweepy.Cursor(api.search, q=hashtag, tweet_mode='extended').items(count): tweets.append({ 'Date': tweet.created_at, 'User': tweet.user.screen_name, 'Text': tweet.full_text, 'Likes': tweet.favorite_count, 'Retweets': tweet.retweet_count }) return tweets except tweepy.TweepError as e: return {'Error': str(e)}
This function takes a hashtag and a count of tweets to retrieve, providing a list of dictionaries with hashtag-related tweet details.
5. Best Practices for Ethical Data Scraping
5.1. Respecting API Rate Limits
Twitter’s API has rate limits to prevent abuse. Make sure to respect these limits to avoid being blocked. Tweepy automatically handles rate limits, but it’s essential to be aware of them.
5.2. Caching Data to Avoid Overloading
Frequent API requests can lead to overloading. Implement caching mechanisms to store previously fetched data locally. This reduces the need for repeated requests, saving bandwidth and preventing API limitations.
6. Putting the Data to Use
6.1. Analyzing and Visualizing Scraped Data
Once you have scraped Twitter data, the real magic begins. You can use libraries like Pandas and Matplotlib to analyze and visualize the data. For example, you can create histograms of tweet engagement, word clouds of popular hashtags, and timelines of user activity.
6.2. Extracting Insights and Trends
With the analyzed data, you can extract valuable insights. Identify peak engagement times, trending topics, and sentiment patterns. These insights can guide marketing strategies, inform decision-making, and offer unique perspectives.
Conclusion
Scraping Twitter data using Python functions opens doors to a wealth of information waiting to be explored. By harnessing the power of Tweepy and adhering to ethical scraping practices, you can transform raw data into valuable insights. Remember, while data scraping is a powerful tool, it’s essential to use it responsibly and respect the platforms you’re extracting data from. So, dive into the world of Twitter data scraping, and unlock the potential of real-time insights.
In this guide, we’ve only scratched the surface. The world of data scraping is vast and ever-evolving, offering endless opportunities for exploration and discovery. So, equip yourself with the knowledge and tools, and start your journey into the exciting realm of Twitter data scraping with Python. Happy scraping!
Table of Contents
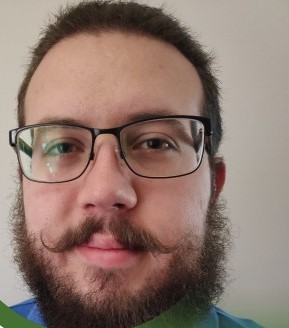
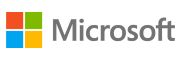