Python Q & A
How to secure a Python web application against threats?
Securing a Python web application is paramount to ensure the safety of your users’ data and maintain the trust of your user base. Here’s a concise guide to enhancing the security of your Python web application:
- Use HTTPS: Ensure all data transmitted between your server and clients is encrypted using SSL/TLS. This protects your users’ data from eavesdropping. You can use tools like Let’s Encrypt to get a free SSL certificate.
- SQL Injection: Use ORMs like SQLAlchemy or Django’s ORM, or if using raw SQL, always use parameterized queries. This will prevent malicious users from injecting harmful SQL commands into your database.
- XSS (Cross-Site Scripting): Never trust user input. Always sanitize and escape data before rendering it on your web pages. Most modern web frameworks, like Django and Flask, offer built-in tools to handle this.
- CSRF (Cross-Site Request Forgery): Use built-in CSRF protection mechanisms provided by your web framework. This ensures that only legitimate requests are processed by your server.
- Session Management: Use secure and HTTP-only cookies for session management. Ensure session timeouts are implemented and consider using token-based authentication for APIs.
- Dependencies: Regularly update your application’s dependencies using tools like `pip`. Vulnerabilities in outdated packages can be exploited, so stay informed and use tools like `pyup` or `dependabot` to get notified about outdated packages.
- Rate Limiting: Implement rate limiting on your endpoints to prevent DDoS attacks and brute-force attempts.
- Logging and Monitoring: Maintain logs of application activity and monitor them for suspicious activities. Use tools like Sentry for error tracking and ELK stack for logging.
- Password Security: Always hash and salt passwords before storing them. Use established libraries like `bcrypt` or `argon2` to handle this.
- Content Security Policy (CSP): Implement CSP headers to reduce the risk of XSS attacks by controlling which resources can be loaded.
- Regular Audits: Conduct regular security audits and penetration testing to identify and fix potential vulnerabilities.
By following these best practices and staying updated with the latest security research, you can significantly enhance the security posture of your Python web application, safeguarding both your platform and your users.
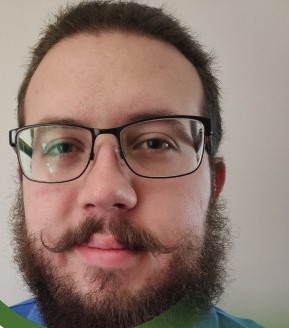
Previously at
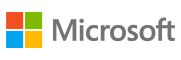
Senior Software Engineer with 7+ yrs Python experience. Improved Kafka-S3 ingestion, GCP Pub/Sub metrics. Proficient in Flask, FastAPI, AWS, GCP, Kafka, Git