How to Use Python Functions for Sentiment Analysis
Sentiment analysis, a vital component of natural language processing (NLP), involves extracting and determining the sentiment expressed in a piece of text, whether it’s positive, negative, or neutral. With the ever-growing volume of text data available on the internet, sentiment analysis has become an essential tool for businesses, researchers, and developers to gain insights into public opinion, customer feedback, and market trends.
Table of Contents
Python, with its rich ecosystem of libraries and tools, provides a robust environment for performing sentiment analysis. In this comprehensive guide, we’ll delve into the world of sentiment analysis using Python functions, breaking down the process step by step, and providing you with code samples and best practices to effectively analyze text sentiment.
1. Introduction to Sentiment Analysis
1.1. What is Sentiment Analysis?
Sentiment analysis, also known as opinion mining, is a technique used to determine the sentiment or emotional tone expressed in a piece of text. It involves analyzing the text and classifying it as positive, negative, or neutral based on the emotions conveyed. This analysis can provide valuable insights into public perception, customer feedback, and overall sentiment trends.
1.2. Applications of Sentiment Analysis
Sentiment analysis has a wide range of applications across various industries:
- Business and Marketing: Companies use sentiment analysis to understand customer opinions about their products and services. This helps them make informed decisions about marketing strategies, product improvements, and customer engagement.
- Social Media Monitoring: Sentiment analysis is extensively used to track and analyze public opinion on social media platforms. It helps organizations gauge the success of their campaigns and identify potential PR crises.
- Financial Analysis: Investors and financial analysts use sentiment analysis to monitor news articles and social media discussions to predict market trends and make investment decisions.
- Political Analysis: Sentiment analysis is employed in political campaigns to understand public sentiment towards different candidates and policies.
- Customer Service: Sentiment analysis can be used to monitor customer feedback and reviews, enabling businesses to identify areas for improvement and address customer concerns promptly.
2. Setting Up Your Environment
2.1. Installing Python and Libraries
Before you start with sentiment analysis, ensure you have Python installed on your system. You can download the latest version of Python from the official Python website.
Next, you’ll need to install the necessary libraries. Two popular libraries for sentiment analysis are NLTK (Natural Language Toolkit) and TextBlob. Install them using the following commands:
python pip install nltk pip install textblob
2.2. Choosing a Sentiment Analysis Library
NLTK and TextBlob are both powerful libraries, but they differ in terms of complexity and capabilities. NLTK provides a comprehensive set of tools for various NLP tasks, including sentiment analysis. On the other hand, TextBlob is built on top of NLTK and provides a simplified API for common NLP tasks, making it a good choice for beginners.
For this guide, we’ll use TextBlob due to its user-friendly interface.
3. Building the Foundation: Text Preprocessing
3.1. Removing Noise from Text
Text data often contains noise such as special characters, punctuation, and HTML tags. Before performing sentiment analysis, it’s crucial to clean the text by removing these elements. You can achieve this using regular expressions or built-in string manipulation functions.
python import re def remove_noise(text): cleaned_text = re.sub(r'<.*?>', '', text) # Remove HTML tags cleaned_text = re.sub(r'[^\w\s]', '', cleaned_text) # Remove punctuation and special characters return cleaned_text
3.2. Tokenization
Tokenization involves splitting a piece of text into individual words or tokens. NLTK provides a convenient method for tokenization.
python from nltk.tokenize import word_tokenize def tokenize(text): tokens = word_tokenize(text) return tokens
3.3. Removing Stopwords
Stopwords are common words that don’t carry significant meaning, such as “the,” “is,” “in,” etc. Removing stopwords can help reduce the noise in your text data.
python from nltk.corpus import stopwords def remove_stopwords(tokens): stop_words = set(stopwords.words("english")) filtered_tokens = [word for word in tokens if word.lower() not in stop_words] return filtered_tokens
3.4. Stemming and Lemmatization
Stemming and lemmatization are techniques to reduce words to their base or root forms. This step helps in normalizing the text and reducing variations in word forms.
python from nltk.stem import PorterStemmer, WordNetLemmatizer def apply_stemming(tokens): stemmer = PorterStemmer() stemmed_tokens = [stemmer.stem(word) for word in tokens] return stemmed_tokens def apply_lemmatization(tokens): lemmatizer = WordNetLemmatizer() lemmatized_tokens = [lemmatizer.lemmatize(word) for word in tokens] return lemmatized_tokens
4. Sentiment Analysis Techniques
4.1. Lexicon-based Approaches
Lexicon-based sentiment analysis involves using a predefined sentiment lexicon or dictionary containing words annotated with sentiment scores. The sentiment score of the text is calculated by aggregating the scores of individual words.
python from textblob import TextBlob def lexicon_sentiment_analysis(text): blob = TextBlob(text) sentiment_score = blob.sentiment.polarity return sentiment_score
4.2. Machine Learning-based Approaches
Machine learning-based sentiment analysis involves training a model on labeled data to predict sentiment. Common algorithms include Naive Bayes, Support Vector Machines, and Neural Networks. You’ll need labeled data for both positive and negative sentiments to train the model.
python from sklearn.feature_extraction.text import CountVectorizer from sklearn.model_selection import train_test_split from sklearn.naive_bayes import MultinomialNB from sklearn.metrics import accuracy_score # Assuming you have labeled data in X (text) and y (sentiment labels) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) vectorizer = CountVectorizer() X_train_vectorized = vectorizer.fit_transform(X_train) X_test_vectorized = vectorizer.transform(X_test) classifier = MultinomialNB() classifier.fit(X_train_vectorized, y_train) predictions = classifier.predict(X_test_vectorized) accuracy = accuracy_score(y_test, predictions)
5. Using Python Functions for Sentiment Analysis
5.1. Defining a Sentiment Analysis Function
Let’s create a function that encapsulates the entire sentiment analysis process, from text preprocessing to sentiment score calculation.
python def analyze_sentiment(text): cleaned_text = remove_noise(text) tokens = tokenize(cleaned_text) filtered_tokens = remove_stopwords(tokens) lemmatized_tokens = apply_lemmatization(filtered_tokens) processed_text = ' '.join(lemmatized_tokens) sentiment_score = lexicon_sentiment_analysis(processed_text) return sentiment_score
5.2. Integrating Text Preprocessing
By encapsulating text preprocessing within the sentiment analysis function, you can streamline the analysis process.
5.3. Applying Lexicon-based Analysis
You can now analyze the sentiment of a given piece of text using the analyze_sentiment function.
python text = "I absolutely loved the movie! The acting was brilliant." sentiment_score = analyze_sentiment(text) if sentiment_score > 0: print("Positive sentiment") elif sentiment_score < 0: print("Negative sentiment") else: print("Neutral sentiment")
5.4. Implementing Machine Learning-based Analysis
To implement a machine learning-based sentiment analysis using the Multinomial Naive Bayes classifier, follow the code snippet provided in the “Machine Learning-based Approaches” section.
6. Best Practices for Accurate Sentiment Analysis
6.1. Choosing the Right Lexicon or Model
The choice of sentiment lexicon or machine learning model depends on your specific use case. Lexicon-based approaches are simpler to implement but may lack accuracy in certain contexts. Machine learning-based models require training data but can provide more accurate results.
6.2. Handling Negations and Context
Negations, such as “not good,” can flip the sentiment of a sentence. Consider the context of negations and modify sentiment scores accordingly.
6.3. Dealing with Slang and Emojis
Slang and emojis can carry significant sentiment but might not be well-captured by lexicons. Consider preprocessing steps to handle slang and emojis or train your machine learning model on data that includes such elements.
7. Putting It All Together: A Sample Project
7.1. Loading and Preprocessing Data
Suppose you have a dataset of customer reviews for a product. Load the data and preprocess the text.
python import pandas as pd # Load the dataset data = pd.read_csv("customer_reviews.csv") # Preprocess the text data['cleaned_text'] = data['review'].apply(remove_noise) data['tokens'] = data['cleaned_text'].apply(tokenize) data['filtered_tokens'] = data['tokens'].apply(remove_stopwords) data['lemmatized_tokens'] = data['filtered_tokens'].apply(apply_lemmatization)
7.2. Choosing the Analysis Approach
Decide whether you want to use lexicon-based or machine learning-based sentiment analysis for your project. Choose the approach that aligns with your data and goals.
7.3. Visualizing the Results
Visualize the sentiment distribution using libraries like Matplotlib or Seaborn.
python import matplotlib.pyplot as plt # Assuming you have sentiment scores in data['sentiment_scores'] plt.hist(data['sentiment_scores'], bins=20, edgecolor='k') plt.xlabel('Sentiment Score') plt.ylabel('Frequency') plt.title('Sentiment Distribution') plt.show()
8. Beyond Sentiment Analysis: Advanced Applications
8.1. Aspect-based Sentiment Analysis
Instead of analyzing overall sentiment, aspect-based sentiment analysis focuses on sentiments related to specific aspects or features of a product or service.
8.2. Sentiment Analysis in Social Media
Social media platforms offer a treasure trove of unstructured text data. Sentiment analysis can help in understanding user opinions, trends, and sentiment shifts in real-time.
8.3. Real-time Sentiment Analysis
Combine sentiment analysis with streaming data to perform real-time sentiment analysis on social media feeds, news articles, and more.
Conclusion
Sentiment analysis is a powerful tool for extracting insights from text data. Using Python functions, you can preprocess text, implement sentiment analysis techniques, and gain valuable insights into public sentiment. By following best practices and exploring advanced applications, you can enhance your sentiment analysis projects and make more informed decisions based on the sentiments expressed in text. Remember, sentiment analysis is a dynamic field, and continuous learning and exploration are key to staying ahead in the realm of natural language processing.
Table of Contents
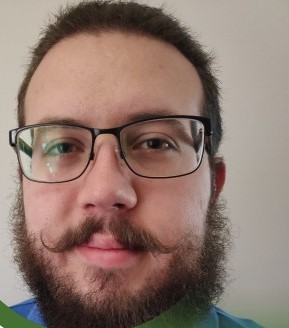
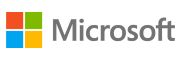